New Moves with Motors
Students program their motors to move to exact positions and for exact degrees.
Questions to investigate
How do robots move precisely?
Prepare
- Ensure SPIKE Prime hubs are charged, especially if connecting through Bluetooth.
- Ensure students have built the Getting Started 2: Motors and Sensors model through step 19 utilized in the Making Moves with Motors Lesson.
1. Engage
Using a tire from the SPIKE Prime set, ask students to think of different ways to measure how far the tire would drive if it went around one rotation. Students should come up with several methods to determine this distance. Prompt student to think about the wheel as a circle. Discuss with them how to calculate the circumference to determine the distance. Ask students how they could measure the circumference of the wheel using a flat surface if they are struggling.
Ask students to imagine they have a robot with wheels. They need to code the motors to move a certain distance. Ask students how could they program the motor to move other than for time? How would this help move the robot more accurately? Discuss ideas with the students about how to move only ½ a turn (0.5 rotation), a full turn (1 rotation), 2 turns (2 rotations).
Note: The wheel has a diameter of 5.6 cm (2.2 in.) and travels 17.6 cm (6.9 in) per rotation or a complete 360o degree circle.
2. Explore
Students will explore how to program motors to move using degrees.
Run Single Motor for Degrees
Running a motor for degrees allows you to determine how far the motor will turn in degree increments – 1/360th of a rotation. Brainstorm with students how they could program the motor to move.
Students should think about what information the software needs to run the hardware correctly. Write a pseudocode program with students for making the motor run for one complete circle.
An example could be:
- Import motor
- Turn motor on
- Move motor 360 degrees
Note: The code written step-by-step does not need to match exactly what will be in the program. Pseudocode is used to help students think through what steps are needed so programming can be coded linearly.
Direct students to open a new project in the Python programming canvas. Ask students to erase any code that is already in the programming area. Students should connect their hub and plug a motor into the A port of their hub.
Provide students with the sample code to move the motor. Ask students to run the program. Students can also copy and paste this code from the Knowledge Base Getting Started 4: Controlling Motors section into the programming canvas.
import motor
from hub import port
import runloop
async def main():
# Run a motor on port A for 360 degrees at 720 degrees per second.
await motor.run_for_degrees(port.A, 360, 720)
runloop.run(main())
Discuss with students how the motor moved. Help students identify the marker on the motor that allows you to set the position to 0 degrees. This will allow students to measure how far the motor moves.
Ask students to set the mark to the 0 position. Run the program again. Ask students if the marker return to the same position.
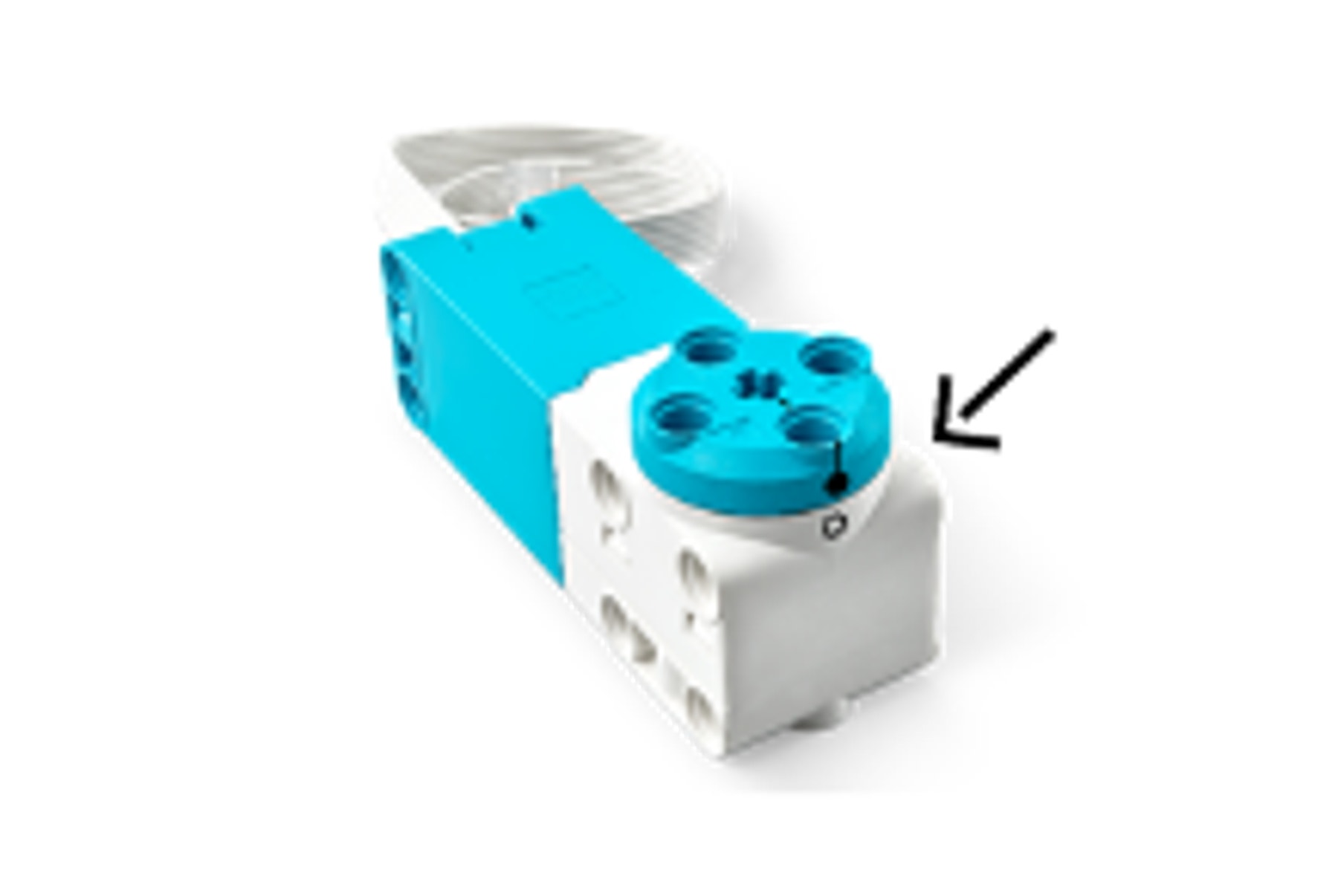
Prompt students to put different numbers into the degree values to see how the motor moves. Have them try large and small numbers.
Ensure students specifically try to run the motor using a negative value. Ask them what changed. (The direction reversed.)
# Run a motor on port A for -360 degrees at 720 degrees per second.
motor.run_for_degrees(port.A, -360, 720)
Ask students to enter a number larger than 360. What happens?
Discuss what students discover after their investigations.
Run Single Motor to Position
Students will explore how to run a single motor to a position.
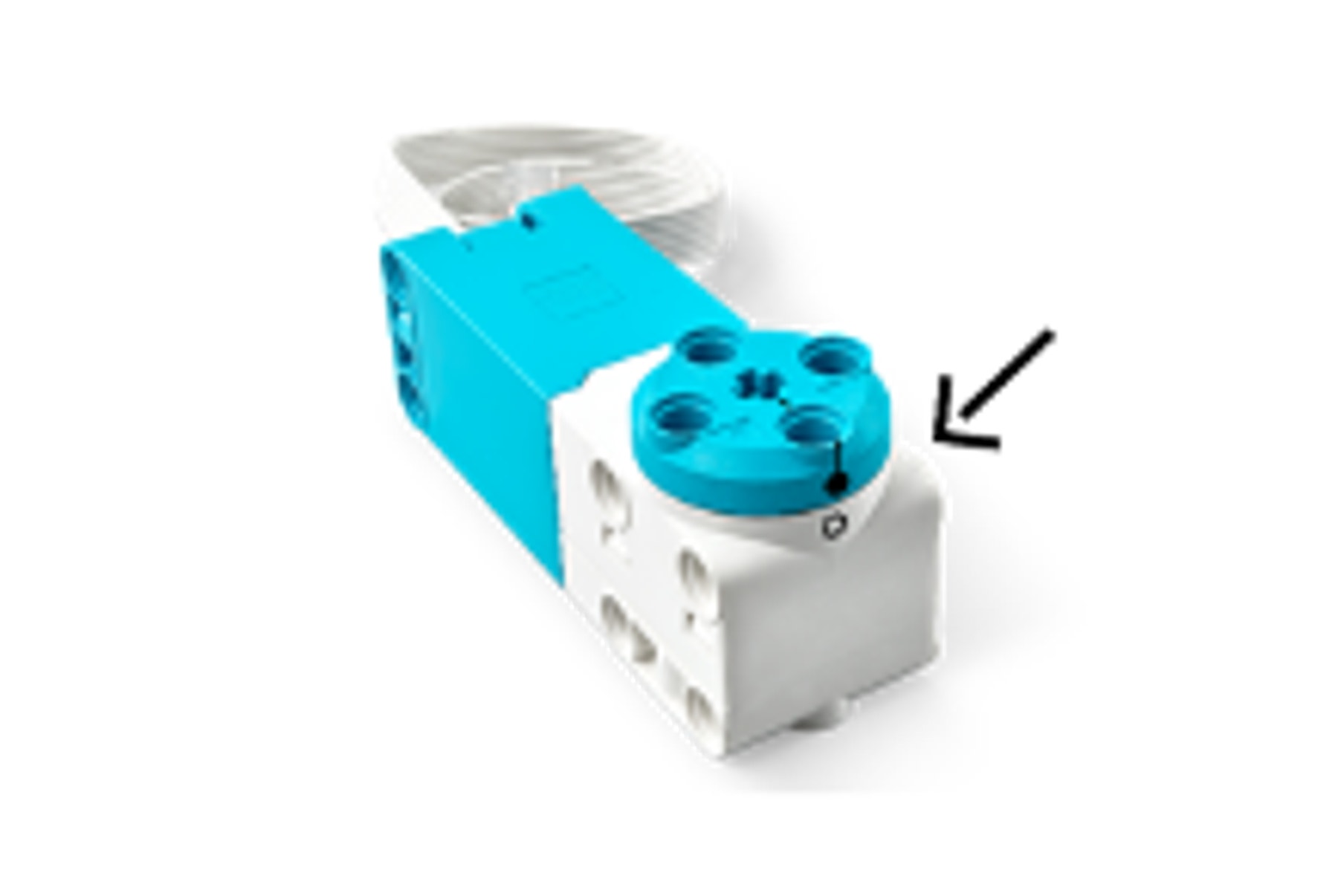
Have students locate the position marker again and ensure it is lined up. When aligned, the motor is in the 0-degree position. Discuss with students how to program the motor to move to a specific position (the motor will stop at an exact position). Prompt students to change their pseudocode to use position instead of degrees.
Provide students with the sample code to move the motor.
import motor
from hub import port
import runloop
async def main():
# Run a motor on port A to 0 degrees at 720 degrees per second.
await motor.run_to_absolute_position(port.A, 0, 720)
runloop.run(main())
Discuss the program with students to identify what happens when they run the code. If students start at the 0 degrees position, the motor will not move.
Ask students to move the motor so it is no longer in the 0 degrees position. Run the program. The motor should move to the 0 degrees position. Which direction did it move to get there?
Ask students to run the program several more times with the motor starting in different positions each time. Discuss what happens. Students should identify that the motor is not moving the same each time. The motor moves in the direction that makes the shortest path back to the 0 degrees position.
Review the lines of code together to highlight why the motor is moving in this way.
What is the longest move a motor can make to return to 0 position?
Ask students to change the code to make the motor stop in different positions. You can provide this sample code to them and then let them explore ideas of how they might change the code.
import motor
from hub import port
import runloop
async def main():
# Run a motor on port A to 0 degrees at 720 degrees per second. Then it will wait 2 seconds and move to 90 degrees in the clockwise direction.
await motor.run_to_absolute_position(port.A, 0, 720)
await runloop.sleep_ms(2000)
await motor.run_to_absolute_position(port.A, 90, 720, direction=motor.CLOCKWISE)
runloop.run(main())
Remind students that when exploring there is a high likelihood of a receiving an error message in the bottom console and the program not executing.
3. Explain
Allow students to share the new example programs they created for degrees and position and discuss ways to program the motor. Discuss how the motor can be set to move for degrees and when this might be useful in a program.
- How does this program work?
- When would it be useful to program a motor to move using degrees or to position?
- Why does using degrees or to position allow for more precision in movement than programming for seconds?
- How did the motor move when you put a number in larger than 360?
- How can you calculate how many degrees you will need to program?
- When using the shortest path in your program for position, why does the motor not move if you start at the 0 degree position?
- How can you program the motor to move in the opposite direction?
4. Elaborate
Work together to work on debugging. Provide students with each of the following codes. Discuss what is wrong with each code. Make sure students run each code and review the error message in the console.
1) What is missing?
import motor
import runloop
async def main():
# Run a motor on port A to 0 degrees at 720 degrees per second. Then it will wait 2 seconds and move to 90 degrees in the clockwise direction.
await motor.run_to_absolute_position(port.A, 0, 720)
await runloop.sleep_ms(2000)
await motor.run_to_absolute_position(port.A, 90, 720, direction=motor.CLOCKWISE)
runloop.run(main())
Students should identify that the port is not imported and therefore cannot set the motor port. The error message in the console is
Traceback (most recent call last):
File "Project 1", line 8, in <module>
File "Project 1", line 6, in main
NameError: name 'port' isn't defined
Students need to add from hub import port
after the import motor line. Have students add this in and run the program again to check their program.
2) What number is wrong and why?
import motor
from hub import port
import runloop
async def main():
# Run a motor on port A to 0 degrees at 720 degrees per second. Then it will wait 2 seconds and move to 90 degrees in the clockwise direction.
await motor.run_to_absolute_position(port.A, 0, 7200)
await runloop.sleep_ms(2000)
await motor.run_to_absolute_position(port.A, 90, 720, direction=motor.CLOCKWISE)
runloop.run(main())
Students should identify that the motor velocity is set to 7200 which is outside the allowable -1000 to 1000 range . The program, however, will still run with no error message in the console. The power will revert to -1000 and run the program.
3) What is wrong?
import motor
from hub import port
import runloop
async def main():
# Run a motor on port A to 0 degrees at 720 degrees per second. Then it will wait 2 seconds and move to 90 degrees in the clockwise direction.
await Motor.run_to_absolute_position(port.A, 0, 720)
runloop.run(main())
Students should recognize that the word Motor is capitalized in line 7. The error message indicates that there is an issue in line 7 and that the error is a value that is not defined.
Traceback (most recent call last):
File "Project 1", line 9, in <module>
File "Project 1", line 7, in main
NameError: name 'Motor' isn't defined
5. Evaluate
Teacher Observation:
Discuss the program with students.
Ask students questions like:
- What are different ways you can program your motors to move?
- Which methods allow for more precise movements?
- What are ways you can program to move using position?
Self-Assessment:
Have students answer the following in their journals:
- What did you learn today that might be helpful in programming robots to move with precision?
- What characteristics of a good teammate did I display today?
- Ask students to rate themselves on a scale of 1-3, on their time management today.
- Ask students to rate themselves on a scale of 1-3, on their materials (parts) management today.
Teacher Support
Students will:
- Program a motor to move to position using the shortest path.
- Program a motor to move to a specific position.
- Program a motor to move a defined number of degrees.
- SPIKE Prime sets
- Devices with the SPIKE App installed.
- Student journals
CSTA
2-CS-02
Design projects that combine hardware and software components to collect and exchange data.
2-AP-10
Use flowcharts and/or pseudocode to address complex problems as algorithms
2-AP-16
Incorporate existing code, media, and libraries into original programs, and give attribution.
2-AP-17
Systematically test and refine programs using a range of test cases.
2-AP-19
Document programs in order to make them easier to follow, test, and debug.
CCSS ELA
SL.8.1
Engage effectively in a range of collaborative discussions (one-on-one, in groups, and teacher-led) with diverse partners on grade 8 topics, texts, and issues, building on others' ideas and expressing their own clearly
SL.8.4
Present claims and findings, emphasizing salient points in a focused, coherent manner with relevant evidence, sound valid reasoning, and well-chosen details; use appropriate eye contact, adequate volume, and clear pronunciation
RST.6-8.3
Follow precisely a multistep procedure when carrying out experiments, taking measurements, or performing technical tasks
L.8.6
Acquire and use accurately grade-appropriate general academic and domain-specific words and phrases; gather vocabulary knowledge when considering a word or phrase important to comprehension or expression
Vocabulary
Degrees
Position