Score!
Apply using conditional statements to keeping score in a game
Questions to investigate
• How can programmers use conditions to keep track of actions or conditions?
Prepare
• Ensure SPIKE Prime hubs are charged, especially if connecting through Bluetooth.
Engage
(Group Discussion, 5 minutes)
Watch the goal lesson engage video available at https://education.lego.com/en-us/lessons/prime-extra-resources/goal#lesson-plan. Ask students to study the way the arms are moving on the player. Students should identify that they move around an entire circle. Discuss with students how they can program the player to score as many goals as possible.
Explore
(Small Groups, 20 minutes)
Students will build a Goal and Player model to investigate different ways to move using the motors.
Direct students to the BUILD section in the SPIKE App. Here students can access the building instruction for Table Top Game. Ask students build the Goal and Accessories and Player models. You can also find the building instructions named Goal! 1 of 2 and Goal! 2 of 2 at https://education.lego.com/en-us/support/spike-prime/building-instructions.
Direct students to open a new project in the Python programming canvas. Ask students to erase any code that is already in the programming area. Students should connect their hub.
Score a Goal
Students will need to program their player to score a goal. Discuss with students the best way to program the motor to move – using seconds, degrees, or for position. Which would be the more efficient way to write your code? Encourage students to try more than one method before settling on their final idea.
Ask students to create a flowchart to document what the program needs to do. If students need help, they should utilize the Knowledge Base and their notes.
Ask students to write and run their program.
Sample Code:
import runloop
import motor
from hub import port
async def main():
# Run a motor on port A to 180 degrees at 720 degrees per second. This will put the arm up to be ready to swing.
await motor.run_to_absolute_position(port.A, 180, 720)
# Run a motor on port A for 360 degrees at 720 degrees per second.
await motor.run_for_degrees(port.A, 360, 720)
runloop.run(main())
Allow students time to investigate and choose the best program. Remind them also to watch the console for any error messages they might receive.
Keeping Score
Students will want to show that they score each time their player makes a goal. Challenge students to add to their previous code to include a conditional statement for keeping score.
Students need to:
• Program the hub to indicate if a goal is scored or not by showing an image, writing a message, or playing a sound. Choose one if a goal is score and different image, word, or sound if a goal is not scored
• Program a way to show the score in the console, adding one if the goal is scored and showing 0 if one is not scored
Brainstorm with students the new lines of code needed through their flowchart. Ask students to update their flowchart to include the new steps in the program.
Flowchart Example:
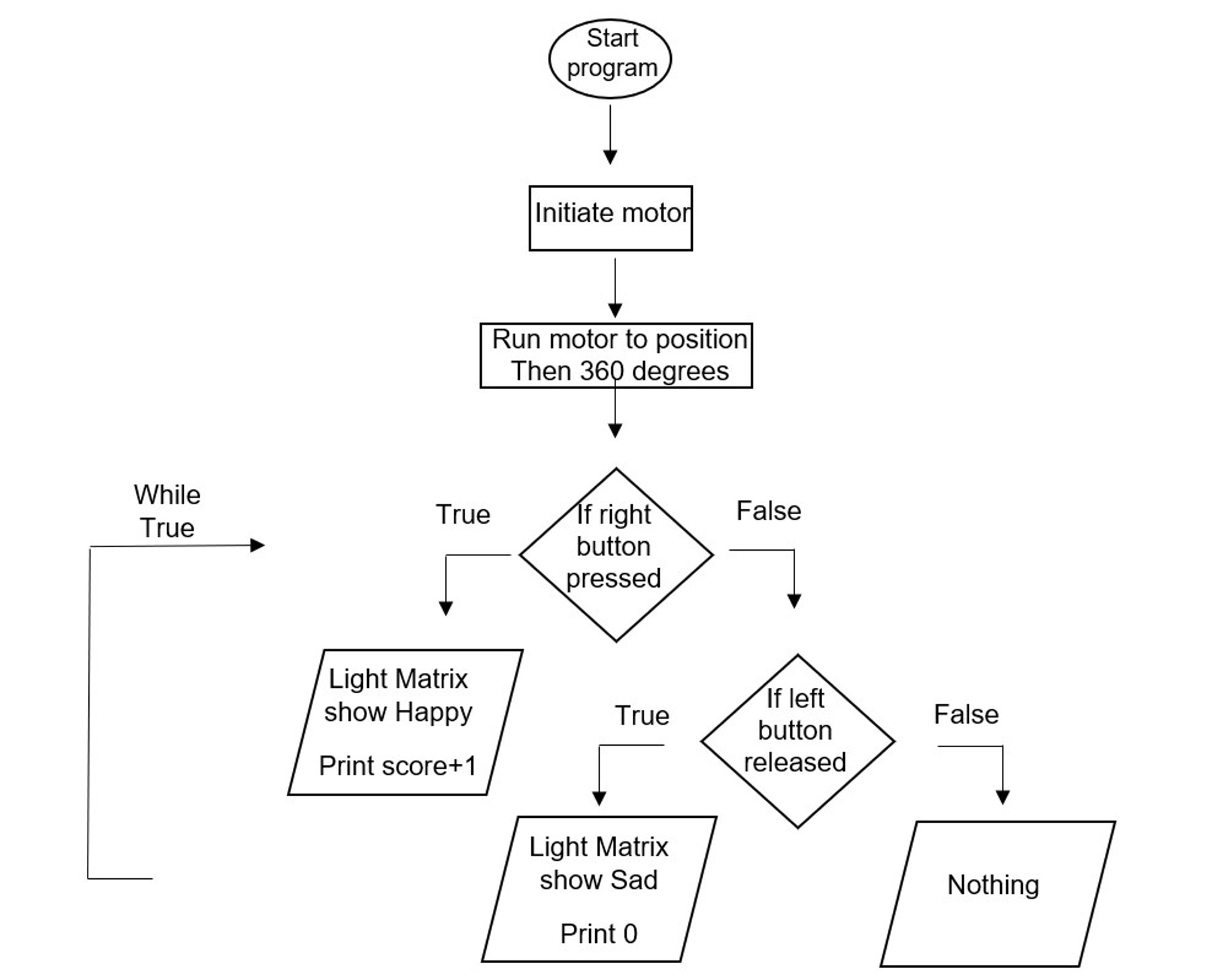
Discuss the flowchart together. Students should notice that we are indicating actions to happen when the conditional statement is both true and false.
Ask students to write and run their program.
Sample Program:
import runloop, motor
from hub import port, light_matrix, button
def right_released():
return not button.pressed(button.RIGHT)
def left_released():
return not button.pressed(button.LEFT)
async def main():
# Run a motor on port A to 180 degrees at 720 degrees per second. This will put the arm up to be ready to swing.
await motor.run_to_absolute_position(port.A, 180, 720)
# Run a motor on port A for 360 degrees at 720 degrees per second.
await motor.run_for_degrees(port.A, 360, 720)
#set the score to 0.
score = 0
#Loop conditional statement to indicate score
while True:
if button.pressed(button.RIGHT):
await runloop.until(right_released)
light_matrix.show_image(light_matrix.IMAGE_HAPPY)
score += 1
print(score)
elif button.pressed(button.LEFT):
await runloop.until(left_released)
light_matrix.show_image(light_matrix.IMAGE_SAD)
print('0')
runloop.run(main())
Note: In this sample program, students need to set a variable for keeping score. The sample uses the name score for the variable and assigns a 0 value to it.
Allow students to run the program several times to try and score a goal. Review the code together as a group.
Explain
(Whole Group, 5 minutes)
Students should explain the program they create to help the player score a goal, indicated a goal was scored, and how a conditional statement was used.
Ask students questions like:
• How did the program allow for you to indicate when you scored a goal?
• How was the conditional statement used?
• How does the flowchart guide you in creating a program?
• What was difficult about this challenge?
• How does the program decide whether to do the if action or the else action? Can both conditions be used?
Explain to students that the program determines if the conditional statement is true or not. The program will only run that portion of the program. So if it is true, the if will run and the else will be ignored.
Elaborate
(Small Groups, 10 minutes)
Challenge the students to change their program to allow the player to keep score by adding up each scored goal rather than just indicating if one goal is scored.
All students time to brainstorm ideas for changing the program to add an additional point each time the player scores a goal. Prompt students to think about how the action will need to be repeated or continued (i.e. leading them to add a while loop).
Ask students to update their flowchart then modify and test their program.
Allow students time to share their program with the class and show how many goals they were able to score in total.
Evaluate
(Group Exercise, 5 minutes)
Teacher Observation:
Discuss the program with students.
Ask students questions like:
• How do if/else conditional statements work?
• How can you create a conditional statement that allows for two true responses?
• How can one input be substituted for another?
Self-Assessment:
Have students answer the following in their journals:
• What did I learn today about using if/else conditional statements?
• What characteristics of a good teammate did I display today?
• Ask students to rate themselves on a scale of 1-3, on their time management today.
• Ask students to rate themselves on a scale of 1-3, on their materials (parts) management today.
Teacher Support
Students will:
• Program movement and light matrix.
• Apply knowledge of conditional statements.
• SPIKE Prime sets ready for student use
• Devices with the SPIKE App installed.
• Student journals
CSTA
2-CS-02 Design projects that combine hardware and software components to collect and exchange data.
2-AP-10 Use flowcharts and/or pseudocode to address complex problems as algorithms
2-AP-13 Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
2-AP-16 Incorporate existing code, media, and libraries into original programs, and give attribution.
2-AP-17 Systematically test and refine programs using a range of test cases.
2-AP-19 Document programs in order to make them easier to follow, test, and debug.