Make a Safer Safe
Students will Investigate using compound conditional statements with logic operators to create a physically protected place to store information
Questions to investigate
• How can multiple conditions be combined into one single condition?
• How can logic operators be used and outcomes predicted?
Prepare
• Ensure SPIKE Prime hubs are charged, especially if connecting through Bluetooth.
• Ensure students have the Safe Deposit Box model built from the Make it Physically Safe lesson.
Engage
(Group Discussion, 5 minutes)
Engage students in thinking about how to combine multiple ideas into one.
Launch a discussion with students about their favorite things to eat. After students have a minute to think about their own favorites, have students come together in pairs to compare their lists. Ask students to create a Venn Diagram showing their favorite foods.
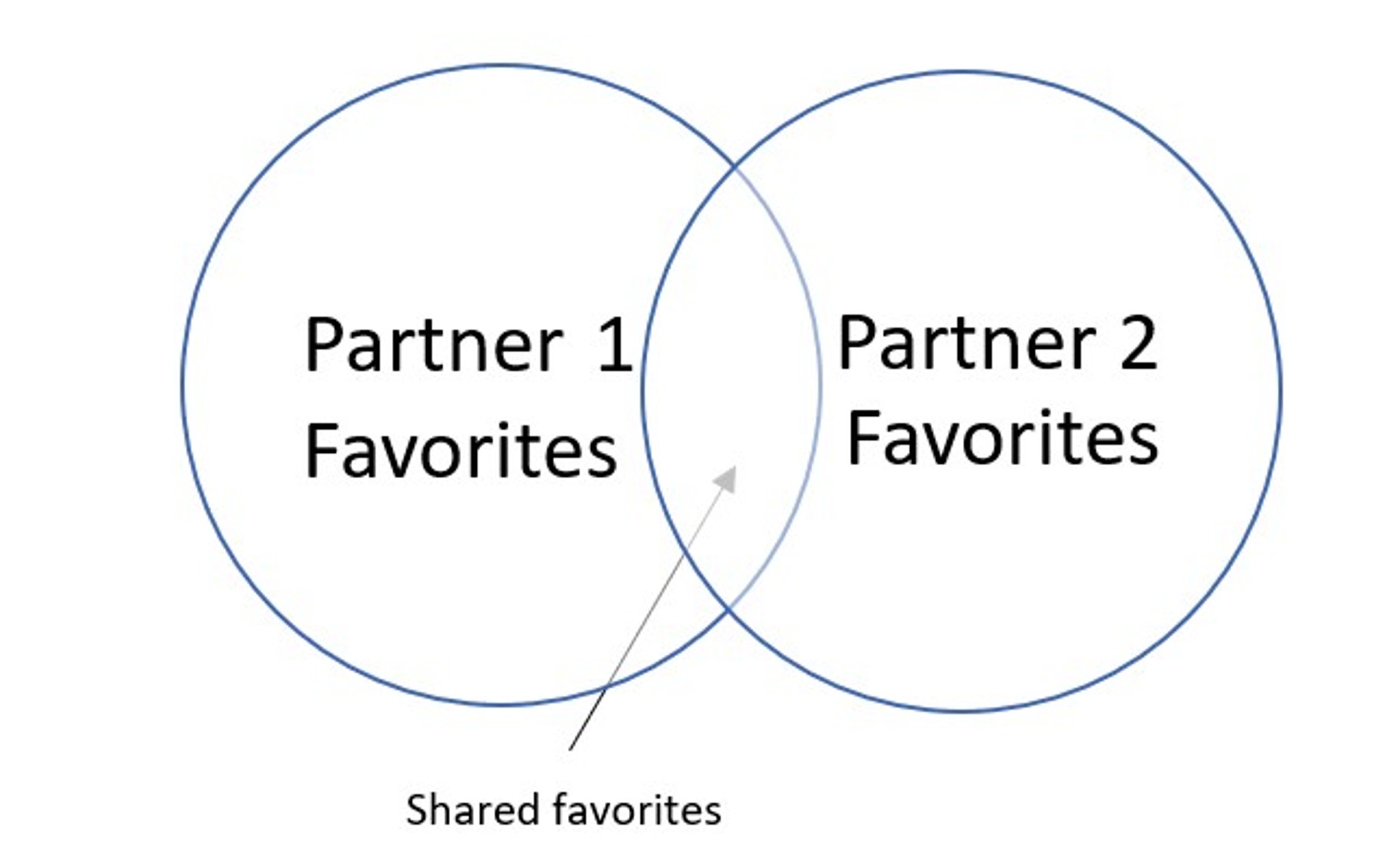
Discuss the Venn Diagrams together. What did the two students have in common? Ask students to consider if they were eating lunch together and had to share, what they would want to eat. Would they want to eat something from one of the individual lists or something they both like?
Discuss students’ ideas together. Have students think about how they could describe what they would eat and not eat encourage the use of the words and, or, and not. For example, I would eat something from my list or the shared list, not the other person’s list.
Explore
(Small Groups, 20 minutes)
Students will investigate combining two conditional statements to create a compound conditional statement using logic operators.
Direct students to the BUILD section in the SPIKE App. Here students can access the building instructions for Super Safe Deposit Box model. Ask students to build the model. If students have the Safe Deposit Box model built from the Make it Physically Safe lesson, then the students will only need to build and attach the arm. The building instructions are also available at https://education.lego.com/en-us/support/spike-prime/building-instructions.
Direct students to open a new project in the Python programming canvas. Ask students to erase any code that is already in the programming area. Students should connect their hub.
Combine Conditional Statements
Have students investigate how the safe works.
Sample program:
from hub import sound, port, light_matrix, button
import app
import runloop
import time
import motor
async def main():
await sound.beep(262, 200)
await sound.beep(523, 200)
#this locks the safe
await motor.run_for_time(port.C, 1000, -500)
await motor.run_to_absolute_position(port.B, 0, 500, stop=motor.COAST)
motor.reset_relative_position(port.B, 0)
await motor.run_to_absolute_position(port.E, 0, 500)
light_matrix.show_image(light_matrix.IMAGE_NO)
#this unlocks the safe
await unlock()
async def unlock():
start_time = time.ticks_ms()
while not button.pressed(button.LEFT) and motor.relative_position(port.B) < 180:
await sound.beep(262, 200)
await motor.run_for_degrees(port.E, 15, 500)
await runloop.sleep_ms(800)
if time.ticks_diff(time.ticks_ms(), start_time) > 5000:
await app.sound.play('Bonk')
return
light_matrix.show_image(light_matrix.IMAGE_NO)
light_matrix.show_image(light_matrix.IMAGE_YES)
await motor.run_to_absolute_position(port.E, 0, 500)
await motor.run_for_time(port.C, 1000, 500)
await app.sound.play('Wand')
runloop.run(main())
Discuss the program together as a group paying attention to how the safe currently locks. Ask students to think about how protected any information in the safe would currently be.
Review the sample program carefully to identify new lines of code. Prompt students to look at the while statement.
if button.pressed(button.LEFT) and not motor.relative_position(port.B) < 180:
Students should recognize that there are two conditional statements being addressed in this line. Introduce the term compound conditional statement to students. Explain that a compound conditional statement is a statement that combines two Boolean expressions. The conditional statement will not be true unless all conditions listed are met.
Explain to students that logic operators are used to combine conditional statements. Point out the two logic operators used in this statement – "and” and “not". “or” is the third type of logical operator.
The program sets two conditions using the and logical operator. Explain to students the word and (lowercase) is used to indicate that both parts of a condition must be true for the entire condition to be true. Also explain that a logic operator allows you to combine more than one Boolean expression or values. These will be evaluated in the program as one Boolean value.
Students will need to type this program into the programming canvas. Students should run the program. Discuss the program together after students run it.
Explain
(Whole Group, 5 minutes)
Discuss with students how the program worked. Ask students questions like:
• How can you combine two conditions into one?
• What are the different types of outcomes that you can have using the and logic operator?
Work together to create a flowchart that explains this program. If one or both conditions are false, then the entire condition is false.
Introduce truth tables to students. These tables are helpful in Boolean Expressions to list all possible outcomes of program using logical operators.
Example Truth Tables:
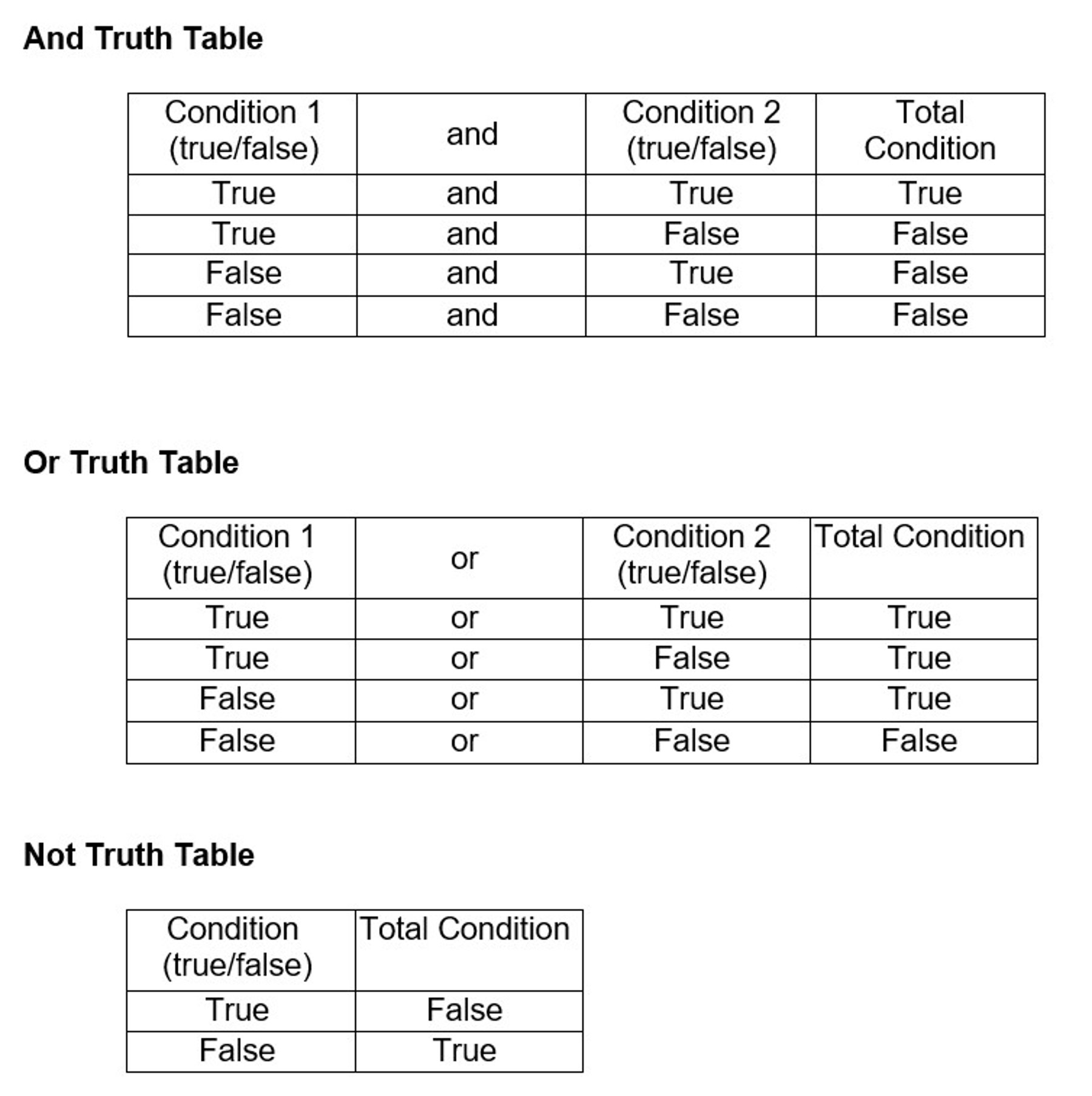
Create a truth table for the sample program. Fill in the possible outcomes based on the combination of how each condition is evaluated. Prompt students to explain why each total condition evaluates as true or false.
Discuss with students how flowcharts and truth tables can be helpful resources when creating programs with combined conditions.
Elaborate
(Small Groups, 10 minutes)
Challenge students to create different conditions to test the logic operator in their conditional statement.
Using their truth table as reference, ask students to try different combinations to create security to test the truth. Students should run their program several times when condition 1 and 2 are changed from true to false in all the combinations in the table to see if the outcomes are all true.
Discuss the outcomes together as a group.
Evaluate
(Group Exercise, 5 minutes)
Teacher Observation:
Discuss the program with students.
Ask students questions like:
• What happened when you changed the logic operators in your program?
• How can truth tables be used to support creating programs with logic operators?
• How does using logic operators allow you to create additional security?
Self-Assessment:
Have students answer the following in their journals:
• What did you learn today about using logic operators and combining two conditions?
• What characteristics of a good teammate did I display today?
• Ask students to rate themselves on a scale of 1-3, on their time management today.
• Ask students to rate themselves on a scale of 1-3, on their materials (parts) management today.
Teacher Support
Students will:
• Investigate using logic operators to combine conditions
• Explore physical security measures
• SPIKE Prime sets ready for student use
• Devices with the SPIKE App installed
• Student journals
CSTA
2-NI-05 Explain how physical and digital security measures protect electronic information.
2-CS-02 Design projects that combine hardware and software components to collect and exchange data.
2-AP-10 Use flowcharts and/or pseudocode to address complex problems as algorithms
2-AP-13 Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
2-AP-16 Incorporate existing code, media, and libraries into original programs, and give attribution.
2-AP-17 Systematically test and refine programs using a range of test cases.
2-AP-19 Document programs in order to make them easier to follow, test, and debug.