Make a Pick and Place Robot
Design, build, and program a robotic system that can pick up an object in one location and place it in another location.
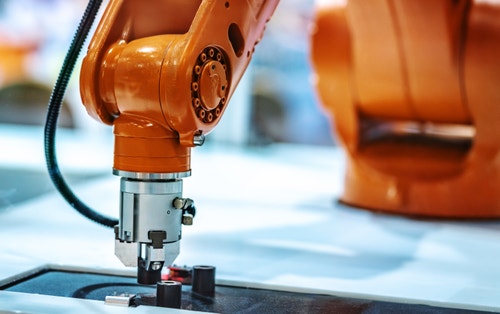
Lesson Plan
Prepare
- Read through this teacher material.
- If you feel it is needed, plan a lesson using the getting started material in the EV3 Lab Software or EV3 Programming App. This will help familiarize your students with LEGO® MINDSTORMS® Education EV3.
Engage (30 Min.)
- Use the ideas in the Ignite a Discussion section below to engage your students in a discussion related to this project.
- Explain the project.
- Split your class into teams of two students.
- Allow your students some time to brainstorm.
Explore (30 Min.)
- Have your students create multiple prototypes.
- Encourage them to explore both building and programming.
- Have each pair of students build and test two solutions.
- Provide them with a large sheet of graph paper and colored pencils or markers.
Explain (60 Min.)
- Ask your students to test their solutions and to choose the best one.
- Make sure they can create their own testing tables.
- Allow some time for each team to finalize their project and to collect assets for documenting their work.
Elaborate (60 Min.)
- Give your students some time to produce their final reports.
- Facilitate a sharing session in which each team presents their results.
Evaluate
- Give feedback on each student’s performance.
- You can use the assessment rubrics provided to simplify the process.
Ignite a Discussion
Pick and place robots are a type of industrial robots that are able to move objects to and from pre-defined places. Based on the shape, weight, and fragility of the objects, different types of grippers can be used to safely and precisely pick the objects up and release them again.
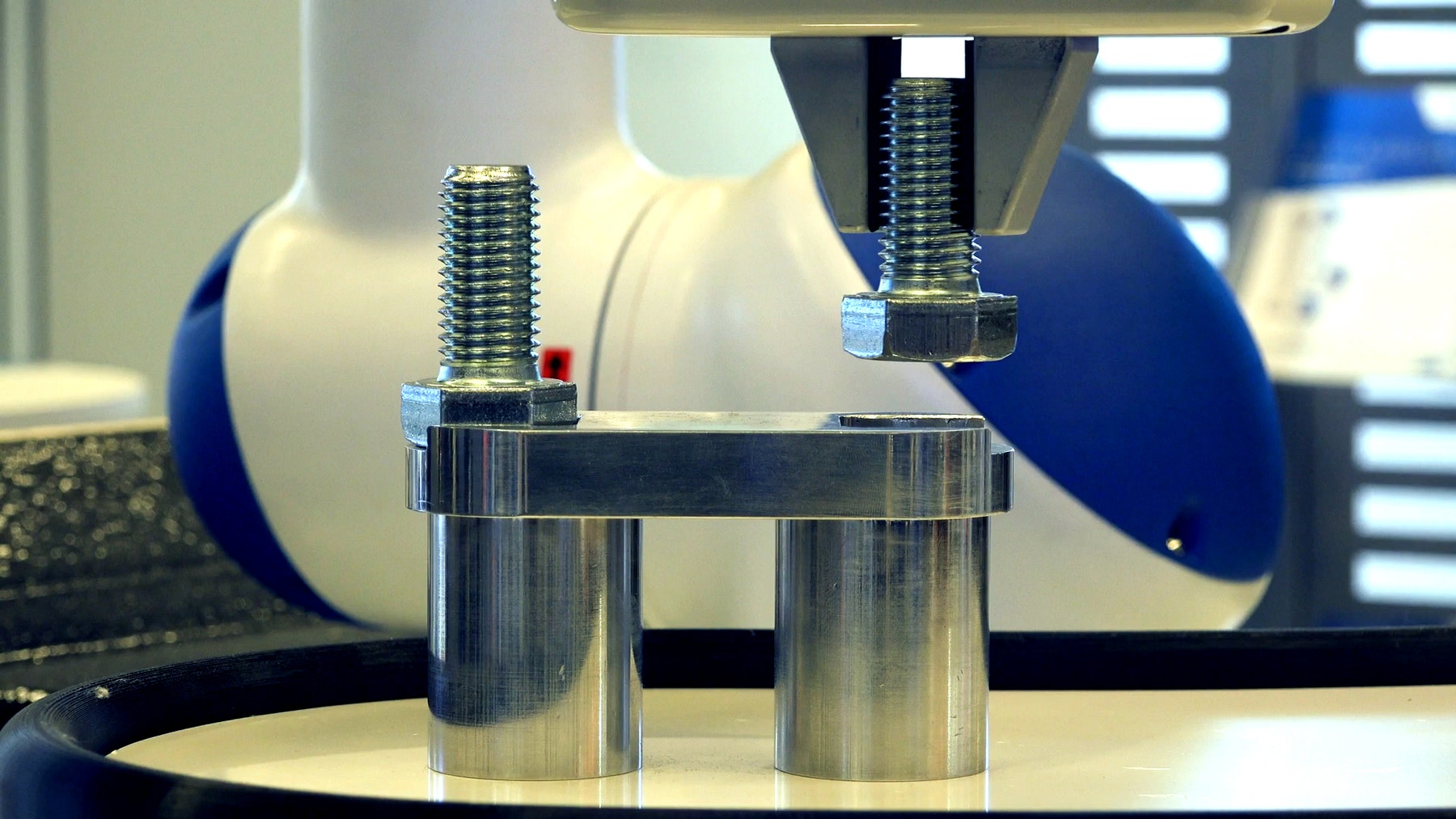
Encourage an active brainstorming process.
Ask your students to think about these questions:
- What is a pick and place robot and where is it used?
- Which type of motorized mechanism can be used to pick up the object?
- How can the robot move the object?
- How can the robot place the object in another location carefully and accurately?
Encourage students to document their initial ideas and explain why they picked the solution they will use for their first prototype. Ask them to describe how they will evaluate their ideas throughout the project. That way, when they are reviewing and revising, they will have specific information they can use to evaluate their solution and decide whether or not it was effective.
Extensions
Language Arts Extension
To incorporate language arts skills development, have your students:
Option 1
- Use their written work, sketches, and/or photos to summarize their design process and create a final report
- Create a video demonstrating their design process starting with their initial ideas and ending with their completed project
- Create a presentation about their program
- Create a presentation that connects their project with real-world applications of similar systems and describes new inventions that could be made based on what they have created
Option 2
To incorporate language arts skills development, have your students:
- Research different machines that prepare medicines and vaccines, and then create a narrative related to working in an automated laboratory creating medicines, framing a benefit and a weakness of automated drug assembly
- From the perspective of data protection, discuss the copyright concerns of potential billion-dollar drug "recipes" being stored in an online system, considering:
- The potential consequences of a company losing drug data
- The benefits of storing sensitive information in online systems
Math Extension
In this lesson, your students created a pick and place robot. As with many automated systems, assessing and improving performance is crucial. Machine learning is a process by which pick and place robots can measure their own performance and make modifications to maintain or improve that performance.
To incorporate math skills development, and explore machine learning, have your students:
- Define the terms accuracy and precision, and apply these definitions to their robotics projects
- Identify the variables that relate to accuracy and precision in their systems (e.g., the speed of their robot may impact its accuracy, precision, or both)
- Set up mini-experiments to test whether their chosen variables impact accuracy, precision, or both
Building Tips
Building Ideas
Give your students an opportunity to build some examples from the links below. Encourage them to explore how these systems work and to brainstorm how these systems could inspire a solution to the Design Brief.
Testing Tips
Encourage your students to design their own tests setup and procedure to select the best solution. These tips can help your students as they set up their test:
- Mark the position of the machine on the graph paper to help ensure that you place it in the same position for each test run.
- Use gridlines to identify 1 cm x 1 cm squares to help in recording the results of each test run.
- Use colored pencils or markers to mark the expected and actual location where the robot places the object.
- Create testing tables to record your observations.
- Evaluate the precision of your robot by comparing the expected results with the actual results.
- Repeat the test at least three times.
Sample Solution
Here is a sample solution that meets the Design Brief criteria:
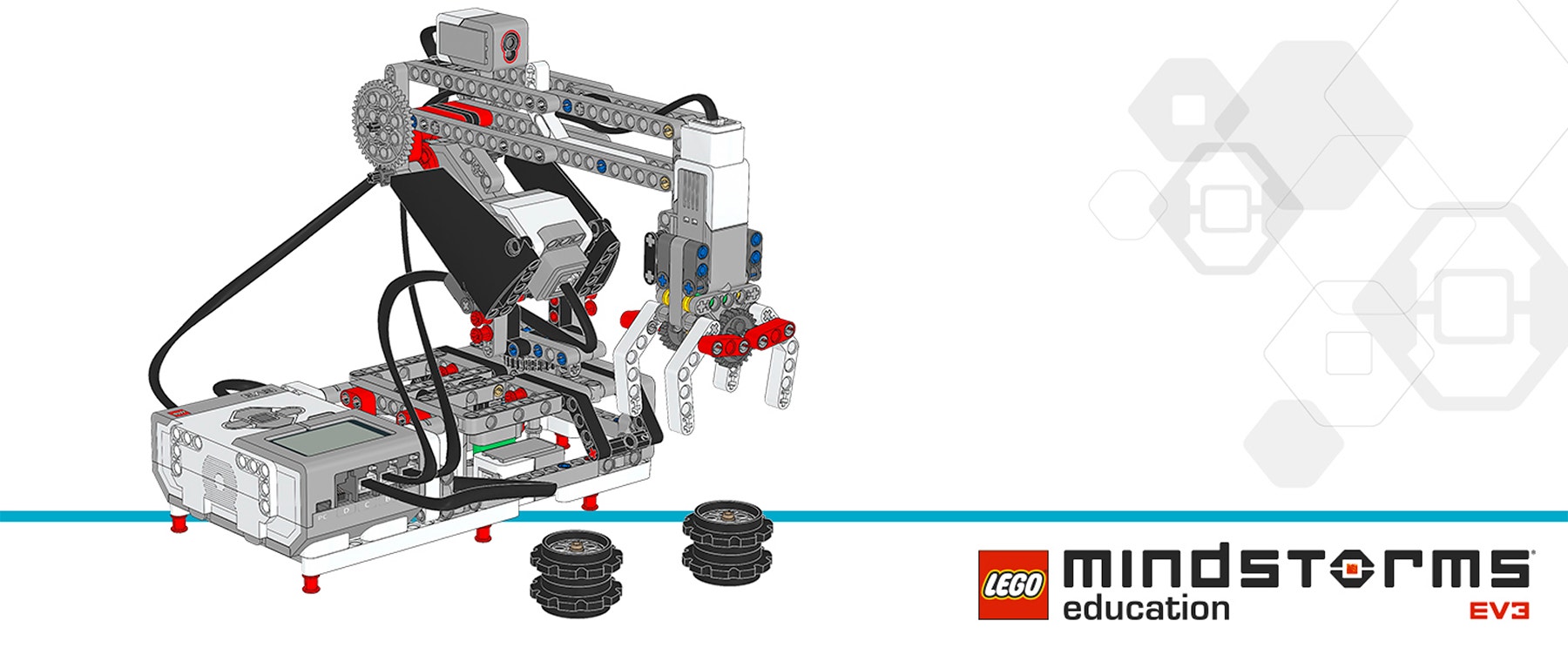
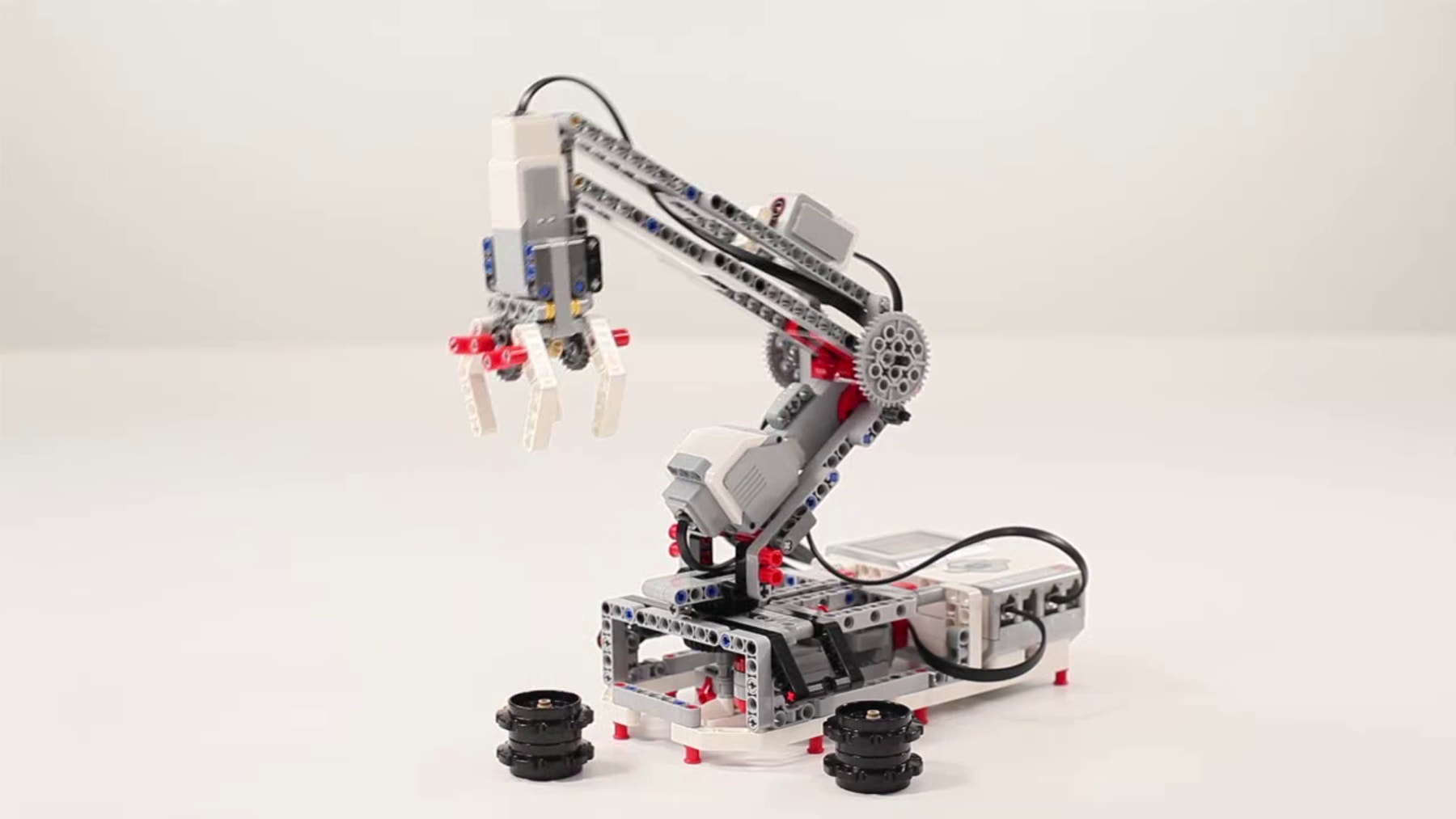
Coding Tips
EV3 MicroPython Sample Program
#!/usr/bin/env pybricks-micropython
from pybricks import ev3brick as brick
from pybricks.ev3devices import Motor, TouchSensor, ColorSensor
from pybricks.parameters import (Port, Stop, Direction, Button,
ImageFile, SoundFile)
from pybricks.tools import wait
# Configure the gripper motor with default settings.
gripper_motor = Motor(Port.A)
# Configure the elbow motor. It has an 8-tooth and a 40-tooth gear
# connected to it. Set the motor direction to counterclockwise, so
# that positive speed values make the arm move upward.
elbow_motor = Motor(Port.B, Direction.COUNTERCLOCKWISE, [8, 40])
# Configure the motor that rotates the base. It has a 12-tooth and a
# 36-tooth gear connected to it. Set the motor direction to
# counterclockwise, so that positive speed values make the arm move
# away from the Touch Sensor.
base_motor = Motor(Port.C, Direction.COUNTERCLOCKWISE, [12, 36])
# Limit the elbow and base accelerations. This results in very smooth
# motion, like that of an industrial robot.
elbow_motor.set_run_settings(60, 120)
base_motor.set_run_settings(60, 120)
# Set up the Touch Sensor. It is used to detect when the base has
# moved to its starting position.
touch_sensor = TouchSensor(Port.S1)
# Set up the Color Sensor. It is used in Reflected Light Intensity
# Mode to detect the white beam when the elbow is in its starting
# position.
color_sensor = ColorSensor(Port.S3)
# Initialize the elbow. This is done by first moving down for 1 second
# and then slowly moving up until the Color Sensor detects the white
# beam. Then the motor stops, holds its position, and resets the angle
# to "0." This means that when it rotates backward to "0" later on, it
# returns to this starting position.
elbow_motor.run_time(-30, 1000)
elbow_motor.run(15)
while color_sensor.reflection() < 30:
wait(10)
elbow_motor.stop(Stop.HOLD)
elbow_motor.reset_angle(0)
# Initialize the base. This is done by first running the base motor
# counterclockwise until the Touch Sensor is pressed. Then the motor
# stops, holds its position, and resets the angle to "0." This means
# that when it rotates backward to "0" later on, it returns to this
# starting position.
base_motor.run(-60)
while not touch_sensor.pressed():
wait(10)
base_motor.stop(Stop.HOLD)
base_motor.reset_angle(0)
# Initialize the gripper. This is done by running the motor forward
# until it stalls. This means that it cannot move any further. From
# this closed gripper position, the motor rotates backward by 90
# degrees, so the gripper opens up. This is the starting position.
gripper_motor.run_until_stalled(200, Stop.COAST, 50)
gripper_motor.reset_angle(0)
gripper_motor.run_target(200, -90)
def robot_pick(position):
# This function rotates the base to the pick up position. There,
# it lowers the arm, closes the gripper, and raises the arm to pick
# up the wheel stack.
base_motor.run_target(60, position, Stop.HOLD)
elbow_motor.run_target(60, -45)
gripper_motor.run_until_stalled(200, Stop.HOLD, 50)
elbow_motor.run_target(60, 0, Stop.HOLD)
def robot_release(position):
# This function rotates the base to the drop-off position. There,
# it lowers the arm, opens the gripper to release the wheel stack,
# and raises the arm again.
base_motor.run_target(60, position, Stop.HOLD)
elbow_motor.run_target(60, -45)
gripper_motor.run_target(200, -90)
elbow_motor.run_target(60, 0, Stop.HOLD)
# Define the 3 destinations for picking up and dropping off the wheel
# stacks.
LEFT = 200
CENTER = 100
RIGHT = 0
# Rotate the base to the center.
base_motor.run_target(60, CENTER, Stop.HOLD)
# This is the main part of the program. It is a loop that repeats
# endlessly.
#
# First, the robot waits until the Up or Down Button is pressed.
# Second, the robot waits until the Center Button is pressed.
# Finally, the robot picks up the wheel stack and drops it off in the
# center.
#
# Then the process starts over, so the robot can pick up another wheel
# stack.
while True:
# Display a question mark to indicate that the robot should await
# instructions.
brick.display.image(ImageFile.QUESTION_MARK)
# Wait until the Up or Down Button is pressed.
while True:
# First, wait until any button is pressed.
while not any(brick.buttons()):
wait(10)
# Then store which button was pressed.
button = brick.buttons()[0]
# If the Up or Down Button was pressed, break out of the loop.
if button in (Button.UP, Button.DOWN):
break
# Play a sound and display an arrow to show where the arm will move.
brick.sound.file(SoundFile.AIR_RELEASE)
if button == Button.UP:
brick.display.image(ImageFile.FORWARD)
elif button == Button.DOWN:
brick.display.image(ImageFile.BACKWARD)
# Wait until the Center Button is pressed, then display a check
# mark to indicate that the instruction has been accepted.
while not Button.CENTER in brick.buttons():
wait(10)
brick.display.image(ImageFile.ACCEPT)
# Pick up the wheel stack. Depending on which button was pressed,
# move left or right.
if button == Button.UP:
robot_pick(RIGHT)
elif button == Button.DOWN:
robot_pick(LEFT)
# Drop off the wheel stack in the center.
robot_release(CENTER)
Career Links
Students who enjoyed this lesson might be interested in exploring these career pathways:
- Information Technology (Computer Programming)
- Manufacturing and Engineering (Machine Technology)
Assessment Opportunities
Teacher Observation Checklist
Create a scale that matches your needs, for example:
- Partially accomplished
- Fully accomplished
- Overachieved
Use the following success criteria to evaluate your students’ progress:
- Students can evaluate competing design solutions based on prioritized criteria and tradeoff considerations.
- Students are autonomous in developing a working and creative solution.
- Students can clearly communicate their ideas.
Self-Assessment
Once your students have collected some performance data, give them time to reflect on their solutions. Help them by asking questions, like:
- Is your solution meeting the Design Brief criteria?
- Can your robot’s movement(s) be made more accurate?
- What are some ways that others have solved this problem?
Ask your students to brainstorm and document two ways they could improve their solutions.
Peer Feedback
Encourage a peer review process in which each group is responsible for evaluating their own and others’ projects. This review process can help students develop skills in giving constructive feedback as well as sharpen their analysis skills and ability to use objective data to support an argument.
Öğretmen Desteği
Students will:
-Use the design process to solve a real-world problem
LEGO® MINDSTORMS® Education EV3 Core Set
Large sheet of graph paper or paper with gridlines
Colored pencils or markers
Main Standards
NGSS
HS-ETS1-2 Design a solution to a complex real-world problem by breaking it down into smaller, more manageable problems that can be solved through engineering.
HS-ETS1-3. Evaluate a solution to a complex real-world problem-based on prioritized criteria and trade-offs that account for a range of constraints, including cost, safety, reliability, and aesthetics, as well as possible social, cultural, and environmental impacts.
CSTA
3A-AP-13 Create prototypes that use algorithms to solve computational problems by leveraging prior student knowledge and personal interests.
3A-AP-17 Decompose problems into smaller components through systematic analysis, using constructs such as procedures, modules, and/or objects.
3A-AP-18 Create artifacts by using procedures within a program, combinations of data and procedures, or independent but interrelated programs.
ISTE Nets
4. Innovative Designer
a. know and use a deliberate design process for generating ideas, testing theories, creating innovative artifacts or solving authentic problems.
b. select and use digital tools to plan and manage a design process that considers design constraints and calculated risks.
c. develop, test and refine prototypes as part of a cyclical design process.
d. exhibit a tolerance for ambiguity, perseverance and the capacity to work with open-ended problems.
5. Computational Thinker
a. formulate problem definitions suited for technology-assisted methods such as data analysis, abstract models and algorithmic thinking in exploring and finding solutions.
c. break problems into component parts, extract key information, and develop descriptive models to understand complex systems or facilitate problem-solving.
6. Creative Communicator
a. choose the appropriate platforms and tools for meeting the desired objectives of their creation or communication.
Extensions Standards
Math Extension
HSS-IC Making Inferences & Justifying Conclusions
Language Arts Extension
CCSS.ELA-LITERACY.W.9-10.1 Write arguments to support claims
CCSS.ELA-LITERACY.W.9-10.2 Write informative/explanatory texts to examine and convey complex ideas, concepts, and information
Öğrenci Materyali
Öğrenci Çalışma Kağıdı
Çevrimiçi bir HTML sayfası veya yazdırılabilir PDF olarak indirin, görüntüleyin veya paylaşın.