Dance to the Beat?
Investigate strategies for debugging programs
Questions to investigate
• How do software engineers identify problems within a program?
Prepare
• Ensure SPIKE Prime hubs are charged, especially if connecting through Bluetooth.
• Ensure students have the Break Dancer model built, which was used in the Break Dancer Break Down lesson.
Engage
(Group Discussion, 5 minutes)
Create a plan for testing programs to identify and fix bugs.
Spark a discussion with students on how to fix problems. Ask students to think of a time when they had an issue or problem or a time that they had an experience that did not turn out as expected.
Discuss what could have helped change the situation. Ask questions like:
• What steps could you have taken upfront to have a better outcome?
• What could you have done when you realized there was a problem to help change the outcome?
Discuss students’ ideas for troubleshooting their experience and list ideas for how to troubleshoot. Share out the steps for identifying bugs or post in the classroom for all students to reference.
Steps to help identify bugs:
• Plan the program by creating a pseudocode or flowchart
• Document the program by using the # for code comments within the program
• Test your program with all types of data that are relevant or expected to be used
• Test your program with types of data that are not expected to be used or outside the range expected
Explore
(Small Groups, 20 minutes)
Students will use a break dancer model to investigate, identifying bugs and fixing them.
Direct students to open a new project in the python programming canvas. Ask students to erase any code that is already in the programming area. If students completed the Break Dancer Break Down lesson, they could start with that program and modify it. Students should connect their hub.
Adding to a Working Program
Students will identify strategies to find bugs when adding to a program.
Ask students to run their existing program from the Break Dancer Break Down lesson or provide students with that program. Running the existing program will allow students to confirm there are no current errors in the program.
Ask students to modify their program so the dancer only moves when the color sensor senses a color according to the following assignment. You can use this chart or work together to create your own.
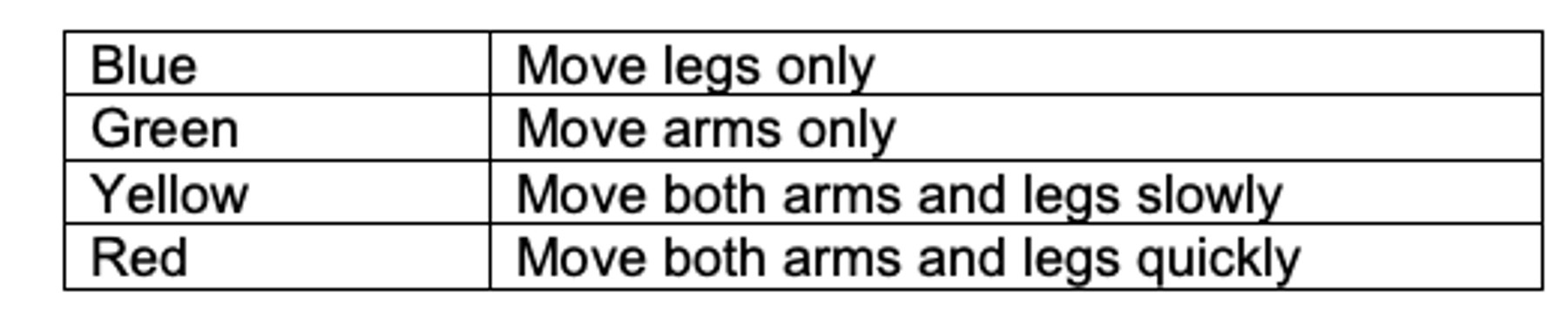
Allow students time to create their new program. Students should test their program to make sure it works. Remind students to utilize the process discussed in the engage section to test the program for bugs.
Ask students to record all debugging activities in their journal. If an error message is received in the console, students can copy this. Students should copy any errors discovered while testing in their journal also.
import runloop
import motor
import color_sensor
import color
from hub import port, light_matrix
async def main():
color_value = color_sensor.color(port.B)
while True:
await runloop.until(lambda: color_sensor.color(port.B) not in (-1, color_value))
color_value = color_sensor.color(port.B)
#if senses blue the arms will move
if color_value == color.BLUE:
light_matrix.show_image(light_matrix.IMAGE_HAPPY)
await motor.run_for_degrees(port.D, 360, 400)
#if senses green the legs will move
elif color_value == color.GREEN:
light_matrix.show_image(3)
await motor.run_for_degrees(port.F, 360, 600)
#if senses yellow both arms and legs will move together slowly
elif color_value == color.YELLOW:
light_matrix.show_image(light_matrix.IMAGE_HAPPY)
motor.run_for_degrees(port.D, 360, 200)
motor.run_for_degrees(port.F, 360, 400)
#if senses red both arms and legs will move together quickly
elif color_value == color.RED:
light_matrix.show_image(light_matrix.IMAGE_HAPPY)
motor.run_for_degrees(port.D, 360, 600)
motor.run_for_degrees(port.F, 360, 800)
#if senses any other color or no color
else:
light_matrix.show_image(light_matrix.IMAGE_SAD)
runloop.run(main())
Explain
(Whole Group, 5 minutes)
Discuss the program with students and where they found bugs.
Ask students questions like:
• What bugs did you find in your program?
• Did you receive any error messages in the console? If so, how was the error message helpful in locating and debugging the program?
• How did you test the program for the expected values? And the unexpected ones?
• Could any of the problems been with the model? Why or why not?
Elaborate
(Small Groups, 10 minutes)
Challenge students to create a program that has their dancer moving to a beat using their color sensor to control movements.
Ask students to utilize their program to create a new dance that has their dancer model moving to a beat. Students can play a song or just hum a beat. The dancer should move fast and slow to the beat which can be controlled when the students hold each color brick up to the color sensor.
Allow students time to investigate the program to see if any changes are needed. Then students should create their movements to match the beat.
Ask students to share their final movements with the class. Consider having a dance party for all the models at the same time.
Evaluate
(Group Exercise, 5 minutes)
Teacher Observation:
Discuss the program with students. Ask students questions like:
• How does testing help identify bugs in your program?
• Why test your program for expected values and unexpected ones?
• What are the types of errors you can receive? How can you use them to fix your program?
Self-Assessment :
Have students answer the following in their journals:
• What did you learn today about identifying and fixing bugs?
• What characteristics of a good teammate did I display today?
• Ask students to rate themselves on a scale of 1-3, on their time management today.
• Ask students to rate themselves on a scale of 1-3, on their materials (parts) management today.
Teacher Support
Students will:
• Identify a problem and debug the program.
• SPIKE Prime sets ready for student use
• Devices with the SPIKE App installed
• Student journals
CSTA
2-AP-10 Use flowcharts and/or pseudocode to address complex problems as algorithms.
2-AP-13 Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
2-AP-17 Systematically test and refine programs using a range of test cases.
2-AP-19 Document programs in order to make them easier to follow, test, and debug.