Counting Your Steps
Investigate using variables and data while counting their steps
Questions to investigate
• How can you add mathematical calculations within a program?
Prepare
• Ensure SPIKE Prime hubs are charged, especially if connecting through Bluetooth.
Engage
(Group Activity, 5 minutes)
Engage students in a conversation about walking and counting their steps. How many students have a device that can do that? How many students use the apps available to them?
Have small groups of students stand shoulder to shoulder and take 5-10 steps forward. Why do they end up at different locations? How would the applications deal with that? Is a step the same for everyone?
Explore
(Small Groups, 20 minutes)
Students will investigate using a smart device to track their steps as they use walking as a mode of transportation.
Direct students to the BUILD section in the SPIKE App. Here students can access the building instructions for the Pedometer model. Students will only need to build the Pedometer and not the stand. Ask students to build the model. The building instructions are also available at https://education.lego.com/en-us/support/spike-prime/building-instructions.
Direct students to open a new project in the Python programming canvas. Ask students to erase any code that is already in the programming area. Students should connect their hub.
Prompt students to think about how to determine how many steps they have taken. Ask students to brainstorm which sensor(s) could be used to determine the number of steps taken. Guide students to the motion sensor. Specifically, have the students think about the roll, pitch, and yaw of the hub.
Discuss the ways the model can move when attached to your body. The pitch of the hub is the hub’s front side tilted forward or backward. (Like tips of the fingers of your outstretched hand tilting up or down.) The roll of the hub is hub’s front side moving down to the right or down to the left. (Like your outstretched hand rolling so the little finger rolls down or up.) The yaw of the hub is the top of the hub moving to the right or left at an angle. (Like turning your outstretched hand so the fingers start pointing forward but move to point to the right or left.)
Students need to create a program that will measure their steps.
Sample Program:
from hub import light_matrix, motion_sensor, button
import runloop
async def main():
step = 0
# reset the yaw angle to 0
motion_sensor.reset_yaw(0)
await light_matrix.write('Walk!')
while True:
# get the tilt values
tilt = motion_sensor.tilt_angles()
# store the yaw value from the tilt tuple
yaw = tilt[0]
if yaw > 75:
step += 1
elif yaw <-75:
step += 1
elif button.pressed(button.LEFT):
await light_matrix.write(str(step))
break
runloop.run(main())
Allow students time to explore the program and try different values.
Introduce some new math functions to students that can be used when creating and comparing their data.
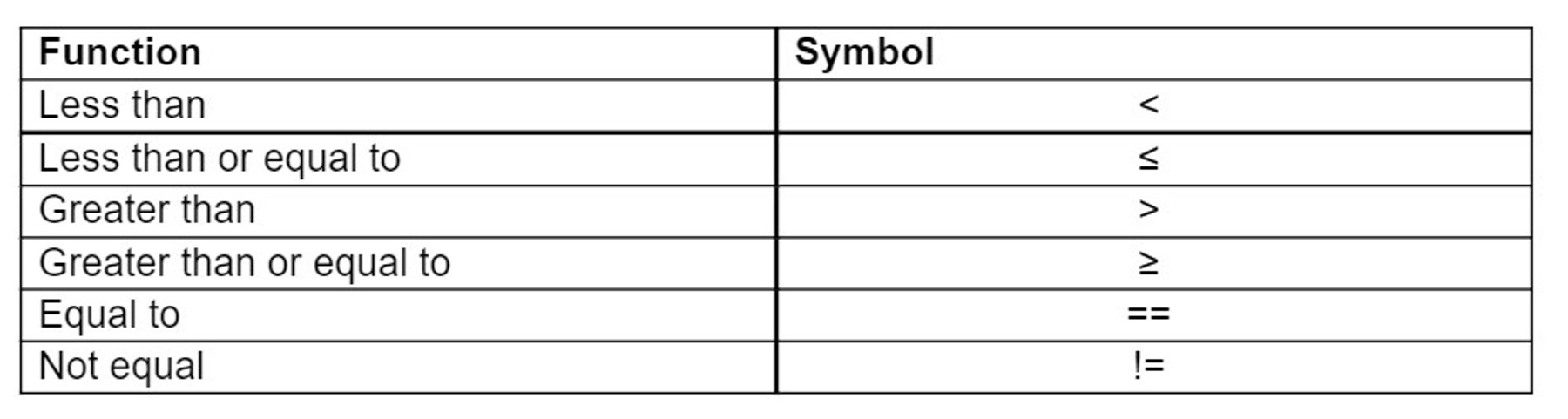
Discuss how these new functions were used in the program to get your steps.
Refining your Program
When getting the yaw angle, the pedometer collected data multiple times per second. The program doesn’t know that the angle is part of your stride. To help, we can add a variable used for the purpose of identifying when the stride so the yaw angle is only collected once which will add only one step for the students.
Sample Program:
from hub import light_matrix, motion_sensor, button
import runloop
import , time
def time_since(some_time):
return time.ticks_diff(time.ticks_ms(), some_time)
async def main():
# define your variables
step = 0
true_step = False
await light_matrix.write('Walk!')
while True:
# get the tilt values
tilt = motion_sensor.tilt_angles()
# store the yaw value from the tilt tuple
yaw = tilt[0]
if yaw > 75 and true_step is False:
step += 1
true_step = True
elif yaw <-75 and true_step is True:
step += 1
true_step = False
elif button.pressed(button.LEFT):
await light_matrix.write(str(step))
break
runloop.run(main())
Allow students time to explore the program.
Explain
(Whole Group, 5 minutes)
Discuss with students how the program worked.
Ask students questions like:
• What was used to count the steps?
• How accurate is your pedometer? Explain.
• Why do you think the pedometer counted too many steps?
Elaborate
(Small Groups, 10 minutes)
Challenge students to add to the program mathematical computations that will calculate the speed. Remember that speed is measured in distance over time.
While testing a program it is good practice to print information into the console to verify the data is accurate and to make sure the program is running as planned.
Sample Program:
from hub import light_matrix, motion_sensor, button
import runloop
import time
def time_since(some_time):
return time.ticks_diff(time.ticks_ms(), some_time)
async def main():
# define your variables
step = 0
true_step = False
stride = 3
distance_walked = 0
time_walked = 0
speed = 0
start_time = 0
elasped = 0
await light_matrix.write('Walk!')
# start the timer
start_time = time.ticks_ms()
while True:
# get the tilt values
tilt = motion_sensor.tilt_angles()
# store the yaw value from the tilt tuple
yaw = tilt[0]
if yaw > 75 and true_step is False:
step += 1
true_step = True
elif yaw <-75 and true_step is True:
step += 1
true_step = False
elif button.pressed(button.LEFT):
await light_matrix.write(str(step))
break
# stop timer
elasped = time_since(start_time)
# make calculations
distance_walked = step * stride
time_walked = elasped/1000
speed = distance_walked / time_walked
# round the time walked and speed to two decimal places
time_walked = str(round(time_walked, 2))
speed = str(round(speed, 2))
# output the data by casting the variables to a string to add the units
print('You took ' + str(step) + ' steps')
print('Your stride is ' + str(stride) + ' feet')
print('You walked for ' + str(time_walked) + ' seconds')
print('You walked ' + str(distance_walked) + ' feet')
print('Your speed is ' + str(speed) + ' feet per second')
runloop.run(main())
Evaluate
(Group Exercise, 5 minutes)
Teacher Observation:
Discuss the program with students.
Ask students questions like:
• What happened when you added the true_step variable?
• How does the true_step variable keep the motion sensor from collecting a reading?
• What did you learn about programming calculations?
• What iterations can you make to improve your pedometer?
Self-Assessment:
Have students answer the following in their journals:
• What did you learn today about using calculations in your program?
• What characteristics of a good teammate did I display today?
• Ask students to rate themselves on a scale of 1-3, on their time management today.
• Ask students to rate themselves on a scale of 1-3, on their materials (parts) management today.
Teacher Support
Students will:
• Integrate mathematical calculations into their programs using variables
• Create a program that will measure footsteps
• SPIKE Prime sets ready for student use
• Devices with the SPIKE App installed
• Student journals
CSTA
2-CS-02 Design projects that combine hardware and software components to collect and exchange data.
2-AP-10 Use flowcharts and/or pseudocode to address complex problems as algorithms
2-AP-13 Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
2-AP-16 Incorporate existing code, media, and libraries into original programs, and give attribution.
2-AP-17 Systematically test and refine programs using a range of test cases.
2-AP-19 Document programs in order to make them easier to follow, test, and debug.