Roaming Vehicles
Design an autonomous car that is safe enough to drive on the streets.
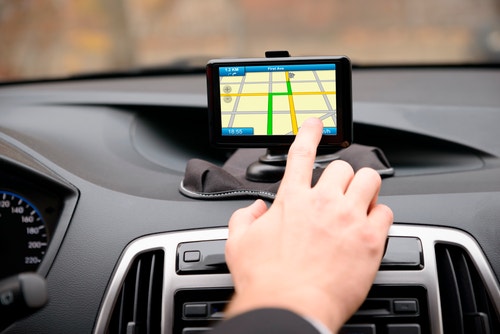
Connect
(5 minutes)
This lesson will focus on arrays and how they can be used to control the actions of the students’ wheeled robots. Students will discover what arrays are, how they work, and why they are important in computer programming. They will also learn how to incorporate an array into their program.
Use the introduction video to ignite a classroom discussion around the following questions:
- How do autonomous cars work?
- What would it take to ensure that autonomous cars are safe?
- What types of movements do autonomous cars need to perform?
- Relate this programming task to programming turn by turn directions from a GPS device. Ask the students to discuss, in groups, how they could program turn by turn directions into their robot to make it move around a course.
Allow the students to select the tool(s) they find most appropriate for capturing and sharing their ideas. Encourage them to document their thoughts using text, videos, images, sketchnotes, or another creative medium.
Construct
(15 to 30 Minutes)
Build
Students will construct the Robot Educator base model.
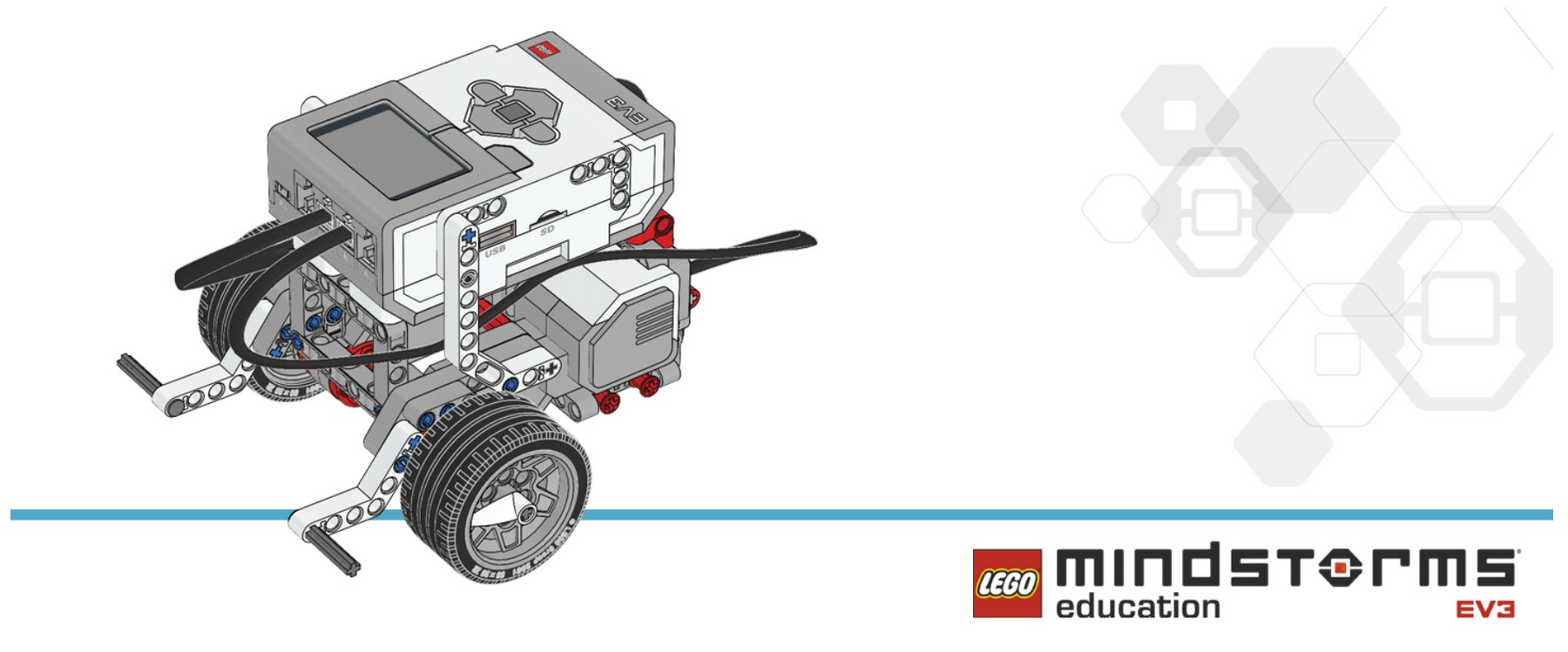
Have the students perform the following building check before they program their robots:
- Are the wires correctly connected from the motors to ports B and C?
- Are the wheels correctly installed?
- Are the wheels rotating freely?
Program
Explain to the students that they are going to program their robot to move according to a recorded set of instructions given to it through the buttons on the EV3 Brick according to these parameters:
- Up Button bumped, the robot moves forward 30 cm
- Down Button bumped, the robot moves backward 30 cm
- Left Button bumped, the robot turns 90 degrees left
- Right Button bumped, the robot turns 90 degrees right
Their program should be divided into two sections: the first is to gather the sequence (e.g., forward, forward, left, forward, right), and the second is to make to robot move according to the sequence.
To start with, they should limit the program to recording one item in the array (an array composed of one item is a variable).
Allow the students to select the tool(s) they find appropriate for capturing and sharing their pseudocode. Encourage them to use text, videos, images, sketchnotes, or another creative medium.
POSSIBLE SOLUTION
FILENAME: CODING-08.EV3 (TAB: 1)
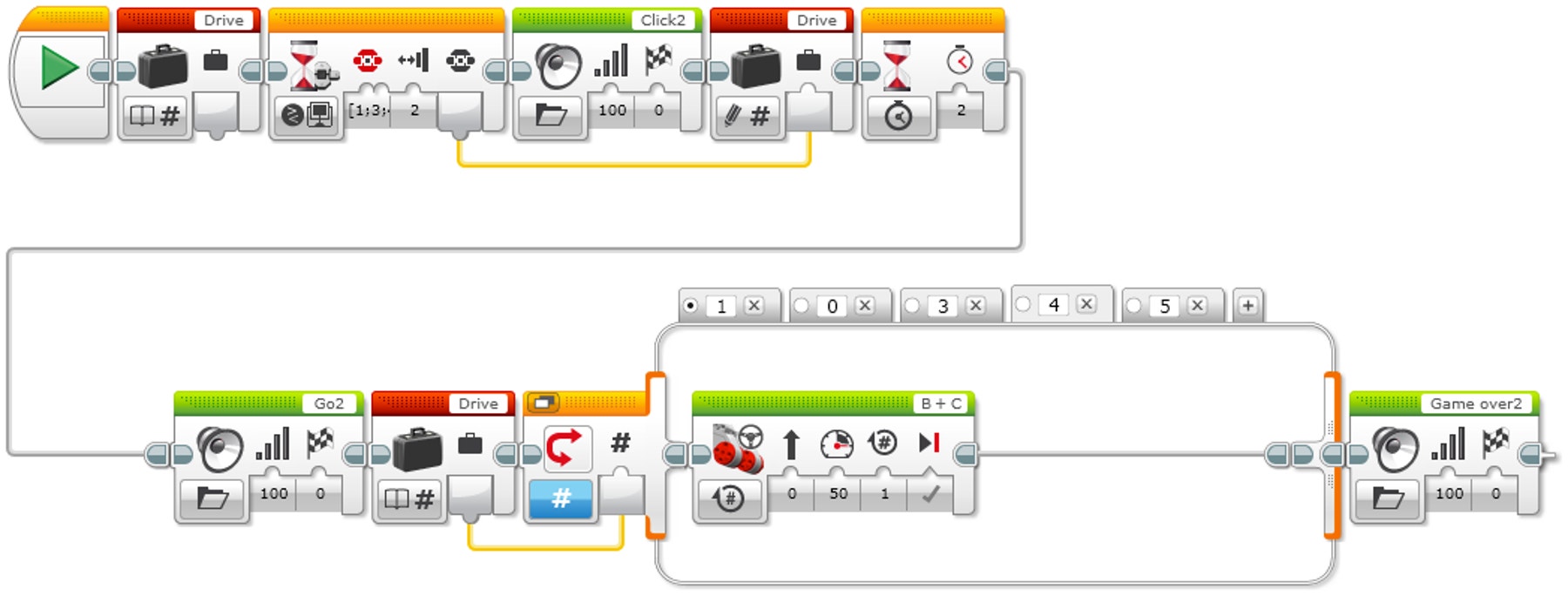
RECORDING ONE ACTION TO MAKE THE ROBOT MOVE
- Start the program.
- Create a Variable Block called “Drive”.
- Wait for a Brick Button to be bumped.
- Play sound “Click 2”.
- Record the numerical value of the pressed button in the variable “Drive”.
- Wait for 2 seconds.
- Play sound “G02”.
- Read the number stored in variable “Drive” and send the value to a switch.
- Numeric switch:
a. If Drive = 1, curve turn the robot left.
b. If Drive = 3, curve turn the robot right.
c. If Drive = 4, move the robot straight forward for 2 rotations of the wheels.
d. If Drive = 5, move the robot straight backward for 2 rotations of the wheels. - Play sound “Game Over 2”.
Note
Refer students to the Robot Educator Tutorials for further assistance. In the EV3 Software:
Robot Educator > Beyond Basics > Arrays
Robot Educator > Tools > My Blocks
Robot Educator > Beyond Basics > Loop
Robot Educator > Beyond Basics > Switch
Robot Educator > Beyond Basics > Variables
Robot Educator > Basics > Straight Move
Robot Educator > Basics > Curved Move
The Array Operations Block is used to store a sequence of data. It is often described as a table consisting of one row with multiple columns.
Contemplate
(35 minutes)
Ask the students to improve their program by using an array to record five actions for their robot.
POSSIBLE SOLUTION
FILENAME: CODING-08.EV3 (Tab: 2)
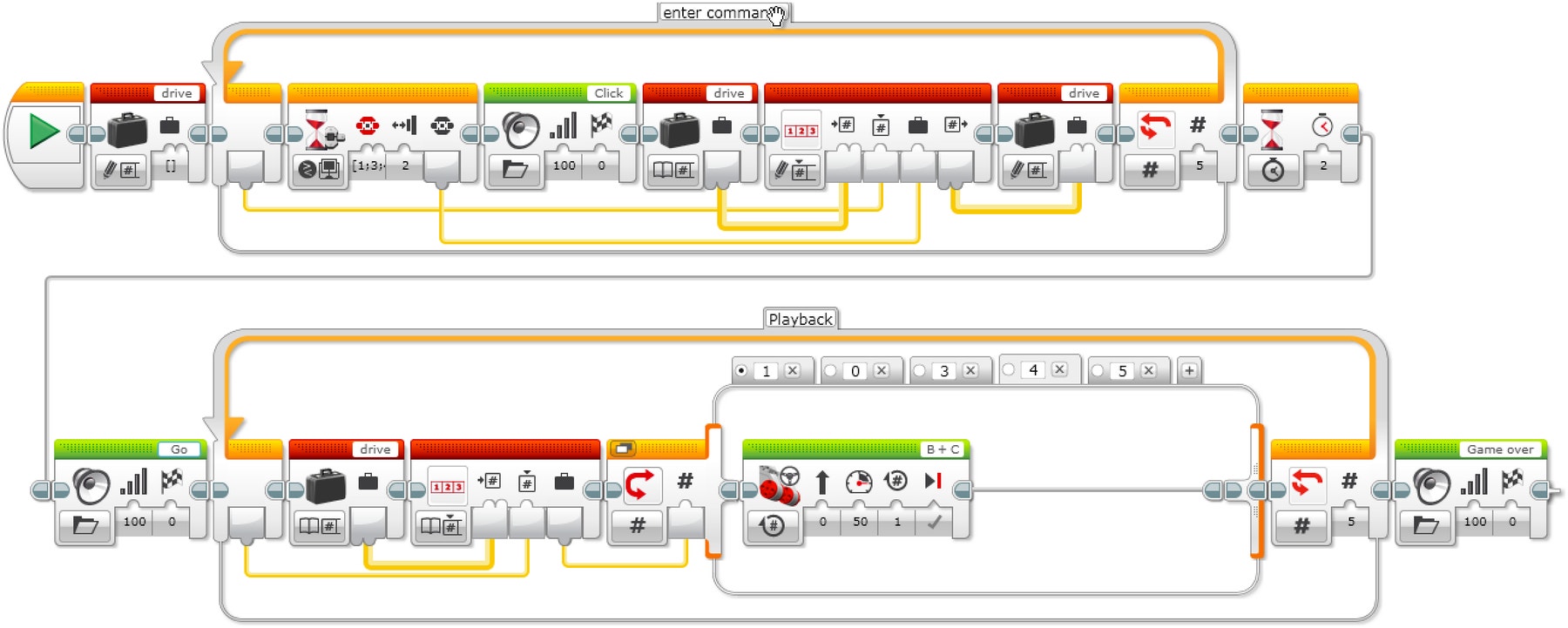
POSSIBLE SOLUTION
FILENAME: CODING-08.EV3 (Tab: 3)
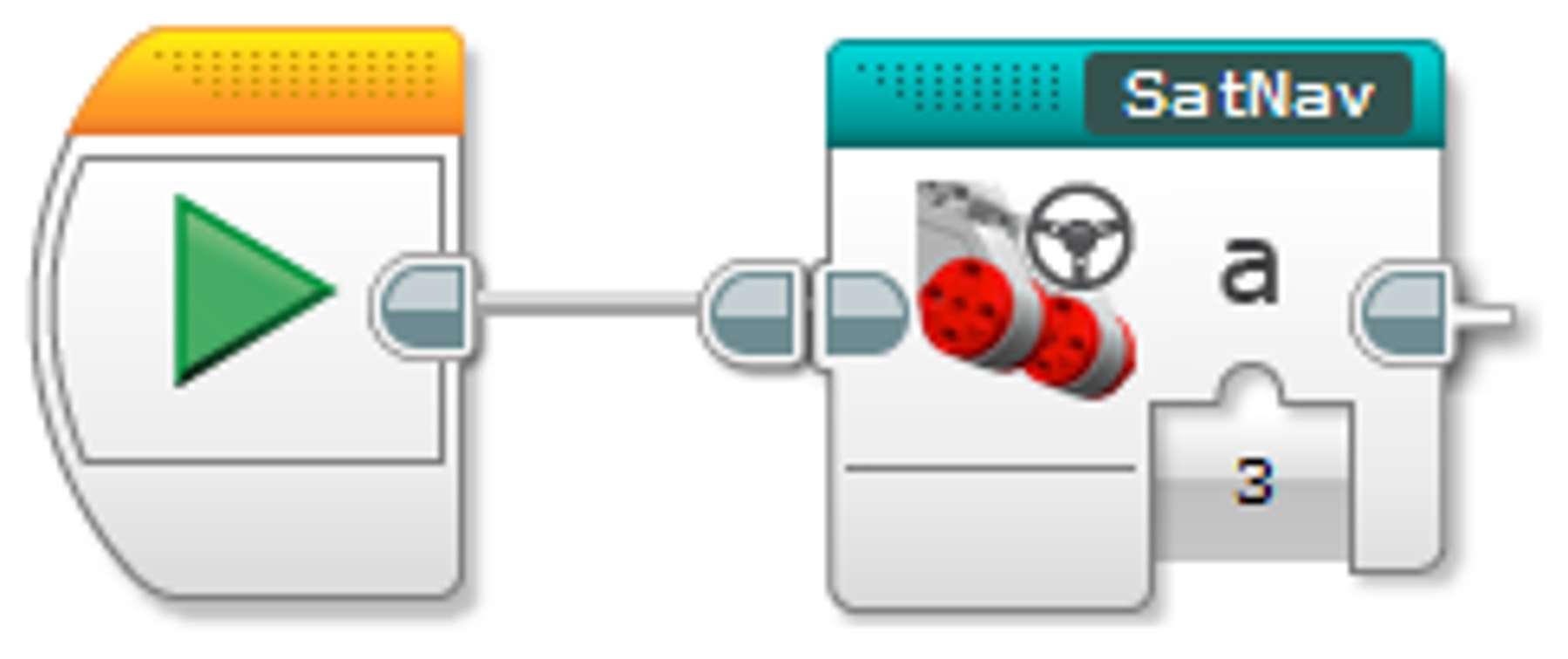
POSSIBLE SOLUTION
FILENAME: CODING-08.EV3 (Tab: SATNAV)
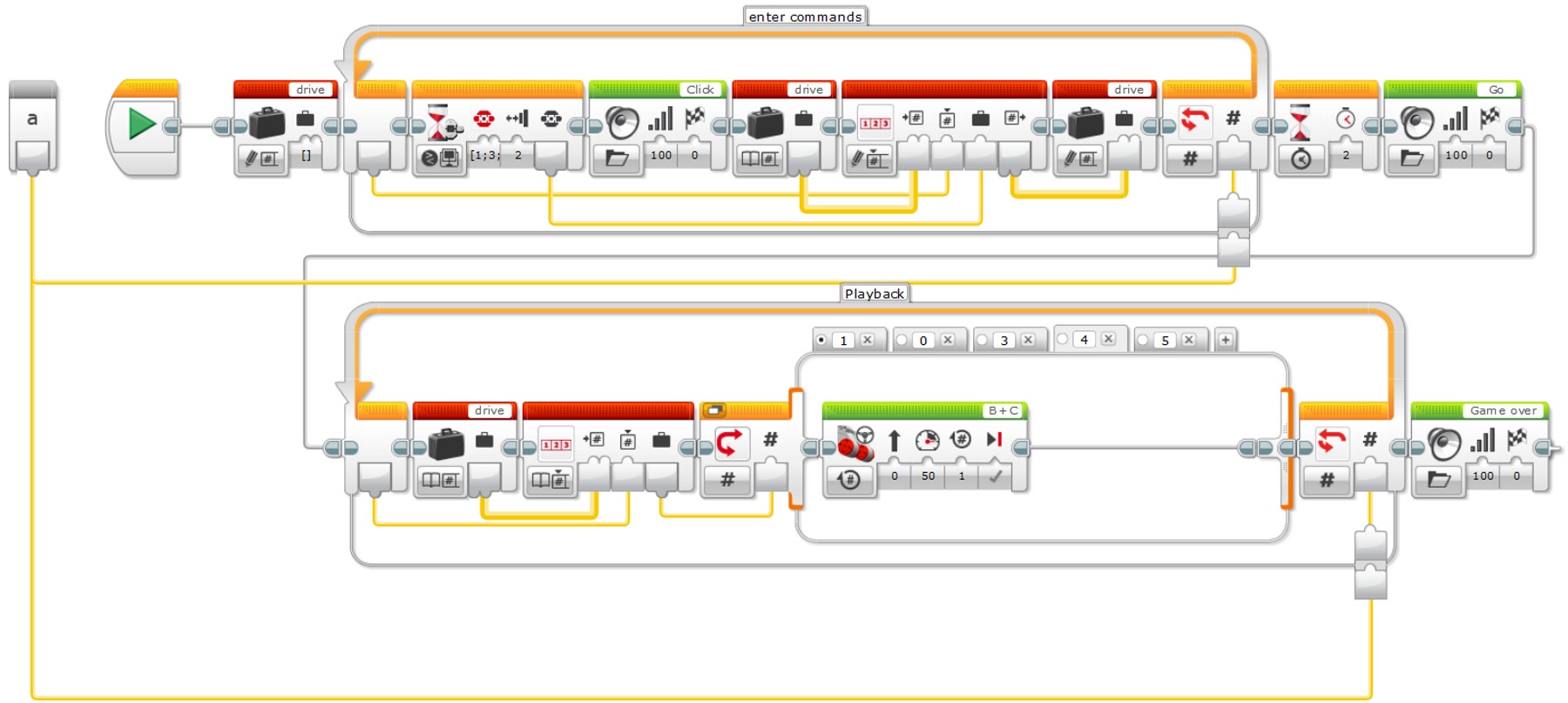
Share
Allow the students to select the tool(s) they find most appropriate for capturing and sharing their creations, unique thinking, and learning process. Encourage them to use text, videos, images, sketchnotes, or another creative medium.
Bring the students together to share their programming successes. Ask how they could have improved their programs.
Does creating a My Block with a parameter make it easier for the students to program the right number of steps?
Ask the students to consider alternative solutions. Can they come up with a new way of programming the robot to move around the room?
Assessment Opportunity
Specific rubrics for assessing computational thinking skills can be found under 'Assessment'.
Continue
(45 Minutes)
Using Text-Based Programming
Have the students explore text-based programming solutions so they can compare different programming languages.
Swift Playground - POSSIBLE SOLUTION
/*
As of summer 2017, there is no way to use the EV3 Brick’s buttons in Swift playground.
In this example, the EV3 up and down buttons have been replaced with touch sensor 1 and touch sensor 2.
The Sound files used in this example are not the same as in the RobotC example
*/
Var drive = 0
repeat
{
ev3.waitFor(seconds: 0.1)
if ev3.measureTouchCount(on: .one) == 1
{
drive = 1
ev3.resetTouchCount(on: .one)
}
else if ev3.measureTouchCount(on : .two) == 1
{
drive = 2
ev3.resetTouchCount(on: .two)
}
} While drive == 0
ev3.waitFor(seconds: 2)
ev3.playSound(file: .start, atVolume: 100, WithStyle: .WaitForCompletion)
if drive == 1
{
ev3. motorOn(forDegrees: 360, on: .c, withPower: 50)
}
else if drive == 2
{
ev3.motorOn(forDegrees: 360, on: .b, withPower: 50)
}
ev3.playSound(file: .goodbye, atVolume: 100, WithStyle: .waitForCompletion)
ROBOT C: POSSIBLE SOLUTION
#pragma config(StandardModel, “EV3 _ REMBOT”)
/*
Use the buttons on the EV3 brick to program the robot to move around.5 commands can be entered into the EV3 brick. ( Left Button = 1, Right Button = 3, Up Button = 4, Down Button = 5)
*/
int drive[5];
task main()
{
for(int i = 0; i < 5; i++) //i = i + 1
{
while(getButtonPress(buttonAny) == 0)
{
//Wait for Any Button to be pressed.
}
if(getButtonPress(buttonLeft) == 1) drive[i] = 1;
else if(getButtonPress(buttonRight) == 1) drive[i] = 3;
else if(getButtonPress(buttonUp) == 1) drive[i] = 4;
else if(getButtonPress(buttonDown) == 1) drive[i] = 5;
playSoundFile(“Click”);
while(bSoundActive)
{
sleep(10);
}
while(getButtonPress(buttonAny) == 1)
{
//Wait for All Buttons to be released.
sleep(10);
}
}
sleep(2000);
playSoundFile(“Go”);
while(bSoundActive)
{
sleep(10);
}
for(int i = 0; i < 5; i++)
{
if(drive[i] == 1)
{
//Turn Left Code.
moveMotorTarget(motorC, 360, 50);
waitUntilMotorStop(motorC);
}
else if(drive[i] == 3)
{
//Turn Right Code.
moveMotorTarget(motorB, 360, 50);
waitUntilMotorStop(motorB);
}
else if(drive[i] == 4)
{
//Forward Code.
moveMotorTarget(motorB, 360, 50);
moveMotorTarget(motorC, 360, 50);
waitUntilMotorStop(motorB);
waitUntilMotorStop(motorC);
}
else if(drive[i] == 5)
{
//Backward Code.
moveMotorTarget(motorB, -360, -50);
moveMotorTarget(motorC, -360, -50);
waitUntilMotorStop(motorB);
waitUntilMotorStop(motorC);
}
}
playSoundFile(“Game over”);
while(bSoundActive)
{
sleep(10);
}
}
Warning! This may be dangerous!
The following is a possible solution using the text-based programming language ROBOTC. You may choose to use any other LEGO MINDSTORMS Education EV3 compatible text-based programming languages.
LEGO Education has no ownership of the ROBOTC platform and does not provide any support or guarantee of the quality of the user experience and technology used. All required set up information is provided by ROBOTC at robotc.net. We recommend always to reinstall the official LEGO MINDSTORMS EV3 Brick firmware when you finish using other programming languages.
Other Program Solutions
Swift Playground - POSSIBLE SOLUTION
/*
As of summer 2017, there is no way to use the EV3 Brick’s buttons in Swift playground.
In this example, the EV3 up and down buttons have been replaced with touch sensor 1 and touch sensor 2.
The Sound files used in this example are not the same as in the RobotC example
*/
var drive = [0, 0, 0, 0, 0]
ev3.resetTouchCount(on: .One)
ev3.resetTouchCount(on: .two)
for i in 0 ... 4
{
repeat
{
ev3.WaitFor(seconds: 0.1)
if ev3.measureTouchCount(on: .one) == 1
{
drive[i] = 1
ev3.resetTouchCount(on : .one)
}
else if ev3.measureTouchCount(on : .two) == 1
{
drive[i] = 2
ev3.resetTouchCount(on: .two)
}
} while drive[i] == 0
ev3.playSound(file: .blip, atVolume: 50, WithStyle: .Wait ForCompletion)
}
ev3.WaitFor(seconds: 2)
ev3.playSound(file: .start, atVolume: 100, WithStyle: .WaitForCompletion)
for i in 0 ... 4
{
if drive[i] == 1
{
ev3.move(forDegrees: 360, leftPort: .b, rightPort: .c, leftPower: 50, rightPower: 50)
}
else if drive[i] == 2
{
ev3.move(forDegrees: 360, leftPort: .b, rightPort: .c, leftPower: -50, rightPower: -50)
}
}
ev3.playSound(file: .goodbye, atVolume: 100, WithStyle: .WaitForCompletion)
ROBOT C: POSSIBLE SOLUTION
Teacher Support
Students will:
Make appropriate use of data structures such as lists, tables and arrays
Extend Boolean logic (such as AND, OR and NOT) and some of its uses in circuits and programming
Use the Variable Block to store information
Use the Array Operations Block
NGSS
MS-ETS1-1. / MS-ETS1-2. / MS-ETS1-3. /MS-ETS1-4.
CSTA
2-A-2-1 / 2-A-7-2 / 2-A-7-3 / 2-A-7-4 / 2-A-5-5 / 2-A-5-6 / 2-A-5-7 / 2-A-4-8 / 2-A-3-9 / 2-A-6-10 / 2-C-7-11 / 2-C-4-12 / 2-D-5-16 / 2-I-1-20
Student Material
Share with:
