Make a Driverless Car
Design an autonomous vehicle that follows user-defined driving commands.
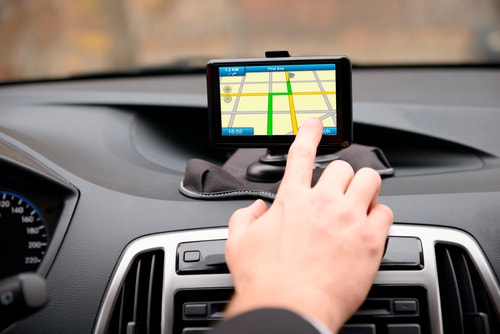
Lesson Plan
Prepare
- Read through this teacher material.
- If you feel it is needed, plan a lesson using the "getting started" material in the EV3 Lab Software or Programming App. This will help familiarize your students with LEGO® MINDSTORMS® Education EV3.
Engage (30 Min.)
- Use the ideas in the Ignite a Discussion section below to engage your students in a discussion related to this project.
- Explain the project.
- Split your class into teams of two students.
- Allow your students some time to brainstorm.
Explore (30 Min.)
- Have your students create multiple prototypes.
- Encourage them to explore both building and programming.
- Have each pair of students build and test two solutions.
Explain (60 Min.)
- Ask your students test their solutions and to choose the best one.
- Make sure they can create their own testing tables.
- Allow some time for each team to finalize their project and to collect assets for documenting their work.
Elaborate (60 Min.)
- Give your students some time to produce their final reports.
- Facilitate a sharing session in which each team presents their results.
Evaluate
- Give feedback on each student's performance.
- You can use the assessment rubrics provided to simplify the process.
Ignite a Discussion
Today, there are many navigation systems used in cars. Some of these systems are now taking on the drivers' responsibility for driving passengers safely to their destinations. Before figuring out the best route between points A and B, autonomous cars must be able to execute a series of movements based on user input.
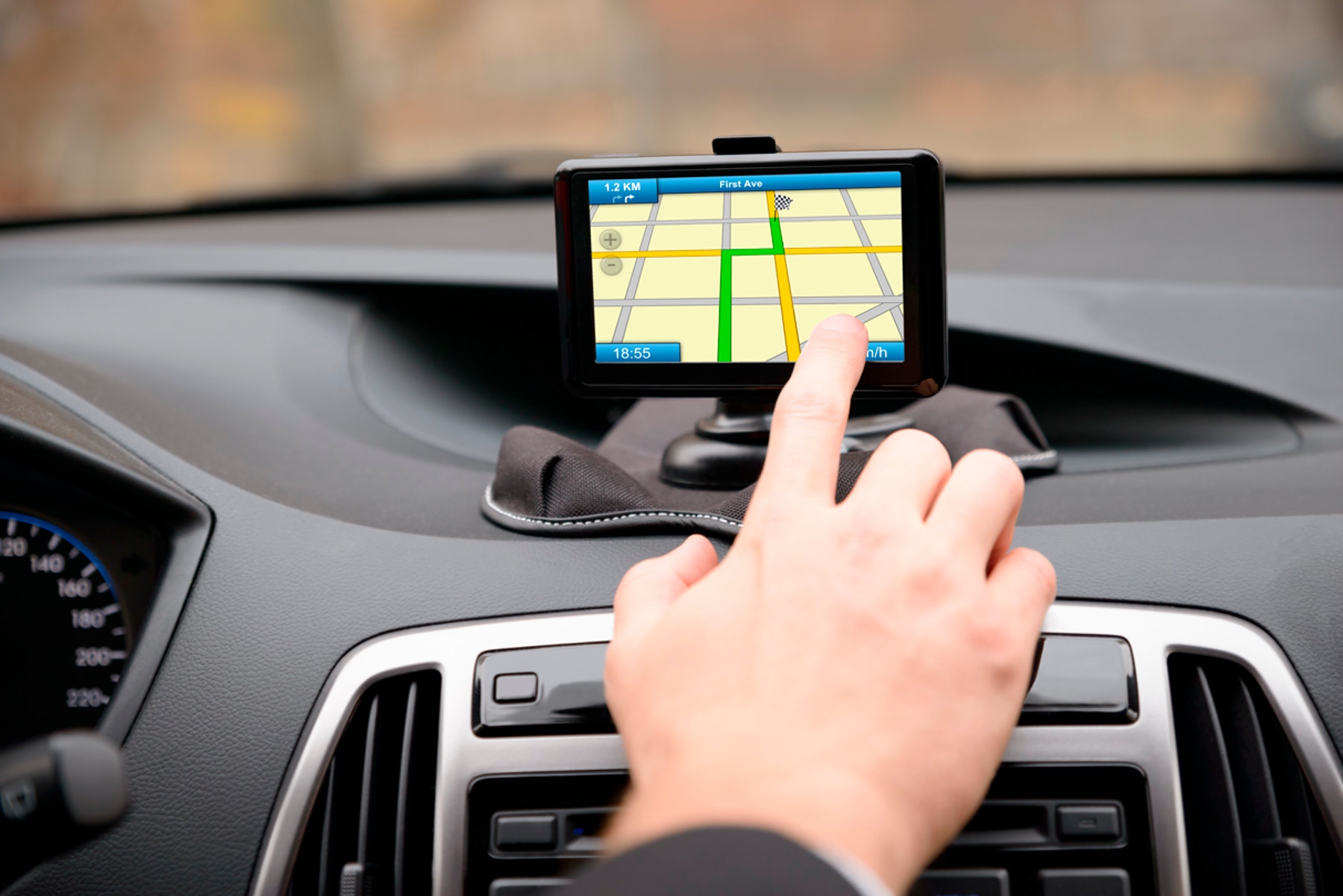
Encourage an active brainstorming process.
Ask your students to think about these questions:
- What are autonomous cars and how do they work?
- Where do autonomous cars get their directions?
- What movements does the car need to perform in order to move through a series of city streets on a north, south, east, west grid?
Give your students some time to answer these questions.
Encourage your students to document their initial ideas and explain why they picked the solution they will use for their first prototype.
Ask them to describe how they will evaluate their ideas throughout the project. That way, when they are reviewing and revising, they will have specific information they can use to evaluate their solution and decide whether or not it was effective.
Pseudocode is a great tool to help students organize their thinking before starting to program.
Building Tips
Start by building a vehicle. Your students can use any of the suggested LEGO MINDSTORMS EV3 Driving Base models or design their own. Make sure there is clear access to the buttons on top of the EV3 Brick. They will be used to control direction in this activity.

Coding Tips
Explain to your students that they are going to program their robot to move according to a recorded set of instructions given to it through the buttons on the EV3 Brick. Use these parameters:
- Up Button bumped, the robot moves forward 30 cm
- Down Button bumped, the robot moves backward 30 cm
- Left Button bumped, the robot turns 90 degrees left
- Right Button bumped, the robot turns 90 degrees right
Recording One Action to Make the Robot Move

Explanation of the Program
- Start the program.
- Create a Variable Block called “Drive.”
- Wait for a brick button to be bumped.
- Play sound “Click 2.”
- Record the numerical value of the pressed button in the variable “Drive.”
- Wait for 2 seconds.
- Play sound “G02.”
- Read the number stored in variable “Drive” and send the value to a Switch.
- Numeric switch:
a. If Drive = 0 (default case), do nothing.
b. If Drive = 1, curve turn the robot left.
c. If Drive = 3, curve turn the robot right.
d. If Drive = 4, move the robot straight forward for 2 rotations of the wheels.
e. If Drive = 5, move the robot straight backward for 2 rotations of the wheels.
- Play sound “Game Over 2.”
Recording Multiple Actions to Make the Robot Move
The Array Operations Block is used to store a sequence of data. It is often described as a table consisting of a single row with multiple columns.

Explanation of the Solution
- Start the program.
- Create a Variable Block called “Drive.” Choose the “WriteNumeric Array” option.
- Create a Loop. The example code is set to run 5 times.
- Wait for a brick button to be bumped.
- Play sound “Click.”
- Read the Variable Block “Drive.” Choose the “Read Numeric Array” option.
- Use the Array Operations Block. Choose “Write at Index - Numeric.”
a. Wire-in the "Drive" Variable Block.
b. Wire the Loop index from the front of the Loop into the index location on the Array Operations Block.
c. Wire the value from the wait for EV3 button block into the value location on the Array Operations Block.
- Write the output of the Array Operations Block into the Variable Block “Drive.”
- Wait for 2 seconds.
- Play sound “Go.”
- Create a second Loop. The example code is set to run 5 times, the same number of times as the first Loop.
- Read the Variable Block “Drive.” Choose the “Read Numeric Array” option.
- Use the Array Operations Block. Choose “Read at Index - Numeric” option.
- Numeric switch:
a. If Drive = 0 (default case), do nothing.
b. If Drive = 1, curve turn the robot left.
c. If Drive = 3, curve turn the robot right.
d. If Drive = 4, move the robot straight forward for 2 rotations of the wheels.
e. If Drive = 5, move the robot straight backward for 2 rotations of the wheels.
- Play sound “Game Over 2.”
Tabs “1” and “2”
EV3 MicroPython Program Solutions
Recording One Action to Make the Robot Move
#!/usr/bin/env pybricks-micropython
from pybricks import ev3brick as brick
from pybricks.ev3devices import Motor
from pybricks.parameters import Port, Stop, Button, SoundFile
from pybricks.tools import wait
from pybricks.robotics import DriveBase
# The Left, Right, Up, and Down Buttons are used to command the robot.
COMMAND_BUTTONS = (Button.LEFT, Button.RIGHT, Button.UP, Button.DOWN)
# Configure 2 motors with default settings on Ports B and C. These
# will be the left and right motors of the Driving Base.
left_motor = Motor(Port.B)
right_motor = Motor(Port.C)
# The wheel diameter of the Robot Educator Driving Base is 56 mm.
wheel_diameter = 56
# The axle track is the distance between the centers of each of the
# wheels. This is 118 mm for the Robot Educator Driving Base.
axle_track = 118
# The Driving Base is comprised of 2 motors. There is a wheel on each
# motor. The wheel diameter and axle track values are used to make the
# motors move at the correct speed when you give a drive command.
robot = DriveBase(left_motor, right_motor, wheel_diameter, axle_track)
# Wait until one of the command buttons is pressed.
while not any(b in brick.buttons() for b in COMMAND_BUTTONS):
wait(10)
# Store the pressed button as the drive command.
drive_command = brick.buttons()[0]
brick.sound.file(SoundFile.CLICK)
# Wait 2 seconds and then play a sound to indicate that the robot is
# about to drive.
wait(2000)
brick.sound.file(SoundFile.GO)
wait(1000)
# Now drive the robot using the drive command. Depending on which
# button was pressed, drive in a different way.
# The robot turns 90 degrees to the left.
if drive_command == Button.LEFT:
robot.drive_time(100, -90, 1000)
# The robot turns 90 degrees to the right.
elif drive_command == Button.RIGHT:
robot.drive_time(100, 90, 1000)
# The robot drives straight forward 30 cm.
elif drive_command == Button.UP:
robot.drive_time(100, 0, 3000)
# The robot drives straight backward 30 cm.
elif drive_command == Button.DOWN:
robot.drive_time(-100, 0, 3000)
# Play a sound to indicate that it is finished.
brick.sound.file(SoundFile.GAME_OVER)
wait(2000)
Recording Multiple Actions to Make the Robot Move
#!/usr/bin/env pybricks-micropython
from pybricks import ev3brick as brick
from pybricks.ev3devices import Motor
from pybricks.parameters import Port, Stop, Button, SoundFile
from pybricks.tools import wait
from pybricks.robotics import DriveBase
# The Left, Right, Up, and Down Buttons are used to command the robot.
COMMAND_BUTTONS = (Button.LEFT, Button.RIGHT, Button.UP, Button.DOWN)
# Configure 2 motors with default settings on Ports B and C. These
# will be the left and right motors of the Driving Base.
left_motor = Motor(Port.B)
right_motor = Motor(Port.C)
# The wheel diameter of the Robot Educator Driving Base is 56 mm.
wheel_diameter = 56
# The axle track is the distance between the centers of each of the
# wheels. This is 118 mm for the Robot Educator Driving Base.
axle_track = 118
# The Driving Base is comprised of 2 motors. There is a wheel on each
# motor. The wheel diameter and axle track values are used to make the
# motors move at the correct speed when you give a drive command.
robot = DriveBase(left_motor, right_motor, wheel_diameter, axle_track)
# Pressing a button stores the command in a list. The list is empty to
# start. It will grow as commands are added to it.
drive_command_list = []
# This loop records the commands in the list. It repeats until 5
# buttons have been pressed. This is done by repeating the loop while
# the list contains less than 5 commands.
while len(drive_command_list) < 5:
# Wait until one of the command buttons is pressed.
while not any(b in brick.buttons() for b in COMMAND_BUTTONS):
wait(10)
# Add the pressed button to the command list.
drive_command_list.append(brick.buttons()[0])
brick.sound.file(SoundFile.CLICK)
# To avoid registering the same command again, wait until the Brick
# Button is released before continuing.
while any(brick.buttons()):
wait(10)
# Wait 2 seconds and then play a sound to indicate that the robot is
# about to drive.
wait(2000)
brick.sound.file(SoundFile.GO)
wait(1000)
# Now drive the robot using the list of stored commands. This is done
# by going over each command in the list in a loop.
for drive_command in drive_command_list:
# The robot turns 90 degrees to the left.
if drive_command == Button.LEFT:
robot.drive_time(100, -90, 1000)
# The robot turns 90 degrees to the right.
elif drive_command == Button.RIGHT:
robot.drive_time(100, 90, 1000)
# The robot drives straight forward 30 cm.
elif drive_command == Button.UP:
robot.drive_time(100, 0, 3000)
# The robot drives straight backward 30 cm.
elif drive_command == Button.DOWN:
robot.drive_time(-100, 0, 3000)
# Play a sound to indicate that it is finished.
brick.sound.file(SoundFile.GAME_OVER)
wait(2000)
Extensions
Language Arts Extension
Option 1
Using Text-Based Programming:
- Have the students explore text-based programming solutions so they can compare different programming languages.
Option 2
In this lesson, your students created a driverless car that operated according to the instructions given to it in an array. What if the driverless cars of the future could be programmed to override input from their human drivers?
To incorporate language arts skills development, have your students:
- Write an argument supporting the claim that driverless cars shouldn't be able to autonomously control their speed in spite of passenger input
- Include specific evidence to support the validity of this claim, citing situational examples where this could leave the passenger at a disadvantage
- Make sure to address the counterclaim that autonomous speed control by driverless cars could be an effective strategy for increasing driver or traffic safety
Math extension
In this lesson, your students created a turn-by-turn sequence of instructions for a driverless car. Using sensors and machine learning, driverless cars can follow instructions and modify the execution of these instructions based on new conditions.
To incorporate math skills development, and explore machine learning applications for driverless cars, give your students a "budget" of a certain number of turns. Then have them:
- Create a grid representing city streets (e.g., five streets that go east to west and five streets that go north to south)
- Pick a starting point and destination · Keeping in mind that the path with the fewest turns represents the best path, analyze three intersections between the starting point and destination
- Determine the probability that their vehicle will get to the destination “under budget” in a random direction
Assessment Opportunities
Teacher Observation Checklist
Create a scale that matches your needs, for example:
- Partially accomplished
- Fully accomplished
- Overachieved
Use the following success criteria to evaluate your students' progress:
- Students can identify the key elements of a problem.
- Students are autonomous in developing a working and creative solution.
- Students can clearly communicate their ideas.
Self-Assessment
Once your students have collected some performance data, give them time to reflect on their solutions. Help them by asking questions, like:
- Is your solution meeting the Design Brief criteria?
- Can your robot’s movement(s) be made more accurate?
- What are some ways that others have solved this problem?
Ask your students to brainstorm and document two ways they could improve their solutions.
Peer Feedback
Encourage a peer review process in which each group is responsible for evaluating their own and others’ projects. This review process can help students develop skills in giving constructive feedback as well as sharpen their analysis skills and ability to use objective data to support an argument.
Career Links
Students who enjoyed this lesson might be interested in exploring these career pathways:
- Business and Finance (Entrepreneurship)
- Manufacturing and Engineering (Pre-Engineering)
Wsparcie dla nauczyciela
Students will:
Use the design process to solve a real-world problem
Main Standards
NGSS
HS-ETS1-2 Design a solution to a complex real-world problem by breaking it down into smaller, more manageable problems that can be solved through engineering.
HS-ETS1-3. Evaluate a solution to a complex real-world problem-based on prioritized criteria and trade-offs that account for a range of constraints, including cost, safety, reliability, and aesthetics, as well as possible social, cultural, and environmental impacts.
CSTA
3A-IC-24 Evaluate the ways computing impacts personal, ethical, social, economic, and cultural practices.
3A-AP-16 Create prototypes that use algorithms to solve computational problems by leveraging prior student knowledge and personal interests.
3A-AP-17 Decompose problems into smaller components through systematic analysis, using constructs such as procedures, modules, and/or objects.
3A-AP-18 Create artifacts by using procedures within a program, combinations of data and procedures, or independent but interrelated programs.
3A-AP-22 Design and develop computational artifacts working in team roles using collaborative tools.
ISTE Nets
4. Innovative Designer
a. know and use a deliberate design process for generating ideas, testing theories, creating innovative artifacts or solving authentic problems.
b. select and use digital tools to plan and manage a design process that considers design constraints and calculated risks.
c. develop, test and refine prototypes as part of a cyclical design process.
d. exhibit a tolerance for ambiguity, perseverance and the capacity to work with open-ended problems.
5. Computational Thinker
a. formulate problem definitions suited for technology-assisted methods such as data analysis, abstract models and algorithmic thinking in exploring and finding solutions.
c. break problems into component parts, extract key information, and develop descriptive models to understand complex systems or facilitate problem-solving.
d. understand how automation works and use algorithmic thinking to develop a sequence of steps to create and test automated solutions.
6. Creative Communicator
a. choose the appropriate platforms and tools for meeting the desired objectives of their creation or communication.
Extensions Standards
Math Extension
HSS-CP Conditional Probability & the Rules of Probability
CCSS.MATH.PRACTICE.MP2 Reason abstractly and quantitatively.
CCSS.MATH.PRACTICE.MP4 Model with mathematics.
Language Arts Extension
CCSS.ELA-LITERACY.W.9-10.1 Write arguments to support claims
Materiały dla uczniów
Arkusz dla ucznia
Pobierz, wyświetl lub udostępnij jako stronę internetową HTML lub plik PDF do wydrukowania.
Udostępnij za pomocą:
