Guess Which Color
Create a game using the color sensors
Questions to investigate
• How can a color sensor be used to provide information to cause reactions?
Prepare
• Ensure SPIKE Prime hubs are charged, especially if connecting through Bluetooth.
Engage
(Group Activity, 5 minutes)
Engage students in thinking about how a color sensor works.
Using 4 different color bricks, challenge students to compete in a game. Tell students you will hold up a color brick and they should do the action assigned to that color. Consider writing the actions listed below somewhere that students can view.
Blue = Clap
Yellow = Jump
Green = Stomp
Red = Spin around
Any other color = do nothing
Ask students to stand up. Play several rounds by holding up different colors and letting the students react. Consider starting slow and then speed up to have the students moving very quickly in the last round.
Discuss this game as a class. Ask students what told them to do a certain action. Discuss how this is similar to a color sensor. The sensor takes in information (input) based on the color it “sees” and will then take the action assigned by the program (or do nothing it that color is not in the code).
Explore
(Small Groups, 20 minutes)
Students will explore working with a color sensor and conditional statement.
Direct students to the BUILD section in the SPIKE App. Here students can access the building instruction for Kiki, the Dog. Students should build the model. You can also find the building instructions at https://education.lego.com/en-us/support/spike-prime/building-instructions listed as Help!
Direct students to open a new project in the Python programming canvas. Ask students to erase any code that is already in the programming area. Students should connect their hub.
If/Else Statements
Students will investigate how to use the color sensor and create an if/else statement.
Introduce students to the Kiki model who has keen sight for different colors using his color sensor “eye”. Prompt students to think about ways to use the color sensor to provide information. Ask students to locate the color sensor they built in the middle of the model. Discuss with students how to create a conditional statement that allows Kiki to play one sound if the blue color is detected and a different sound if the statement is false.
Create a flowchart for this program.
Sample Flowchart:
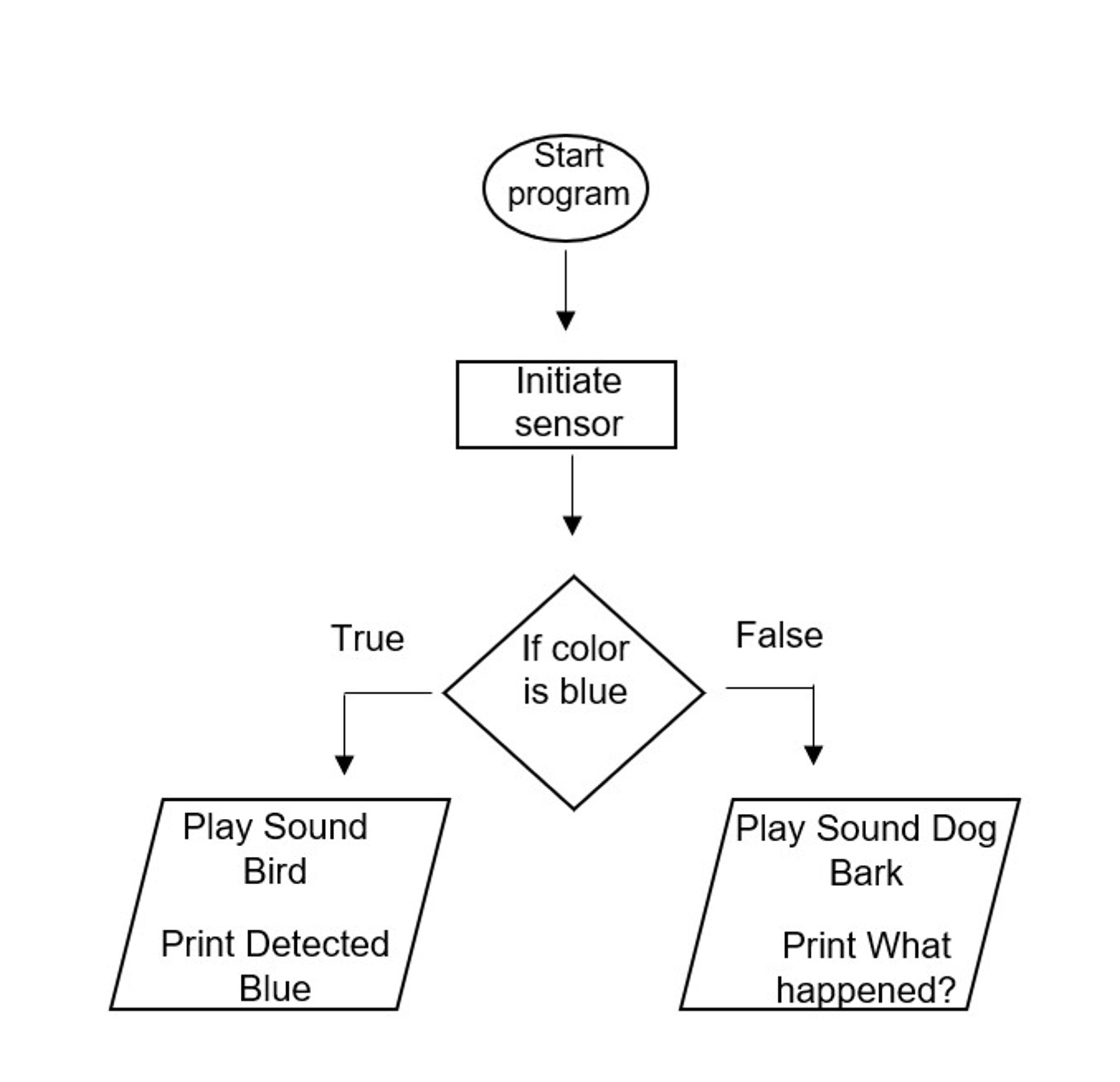
Provide students with this sample code to use the color sensor. Students will need to type this program into the programming canvas. Ask students to run the program.
from hub import port
import runloop
import color_sensor
import color
from app import sound
async def main():
#if the color sensor senses blue, it will play bird sound
if color_sensor.color(port.A) == color.BLUE:
await sound.play('Bird')
print('Blue Detected')
#if the color sensor does not sense blue, it will play dog bark 1 sound
else:
await sound.play('Dog Bark 1')
print('What happened?')
runloop.run(main())
Allow students time to investigate the program including changing the detected color. Discuss the program together as a group.
Explain
(Whole Group, 5 minutes)
Discuss with students how the program worked.
Ask students questions like:
• How does this program work?
• How does the if/else statement work (using true/false language)?
• Why does the model not react to all three colors differently in the program?
• How does the else statement work?
Elaborate
(Small Groups, 10 minutes)
Students will investigate how to combine conditional statements into a single statement using the Elif code.
Prompt students to think about how to add to their program so that we can play different sounds for each color. Ask students to play one sound if blue is detected, a different sound if green is detected, and a third sound if neither of those are detected.
Introduce the elif statement to students. Elif is a combination of else and if and can only be included following an if statement. An elif statement allows the program to set a new condition if the first condition or if statement is read as false, providing a next step in the program.
Share the sample flowchart with students to discuss the path of a program that includes an elif statement.
Sample Flowchart:
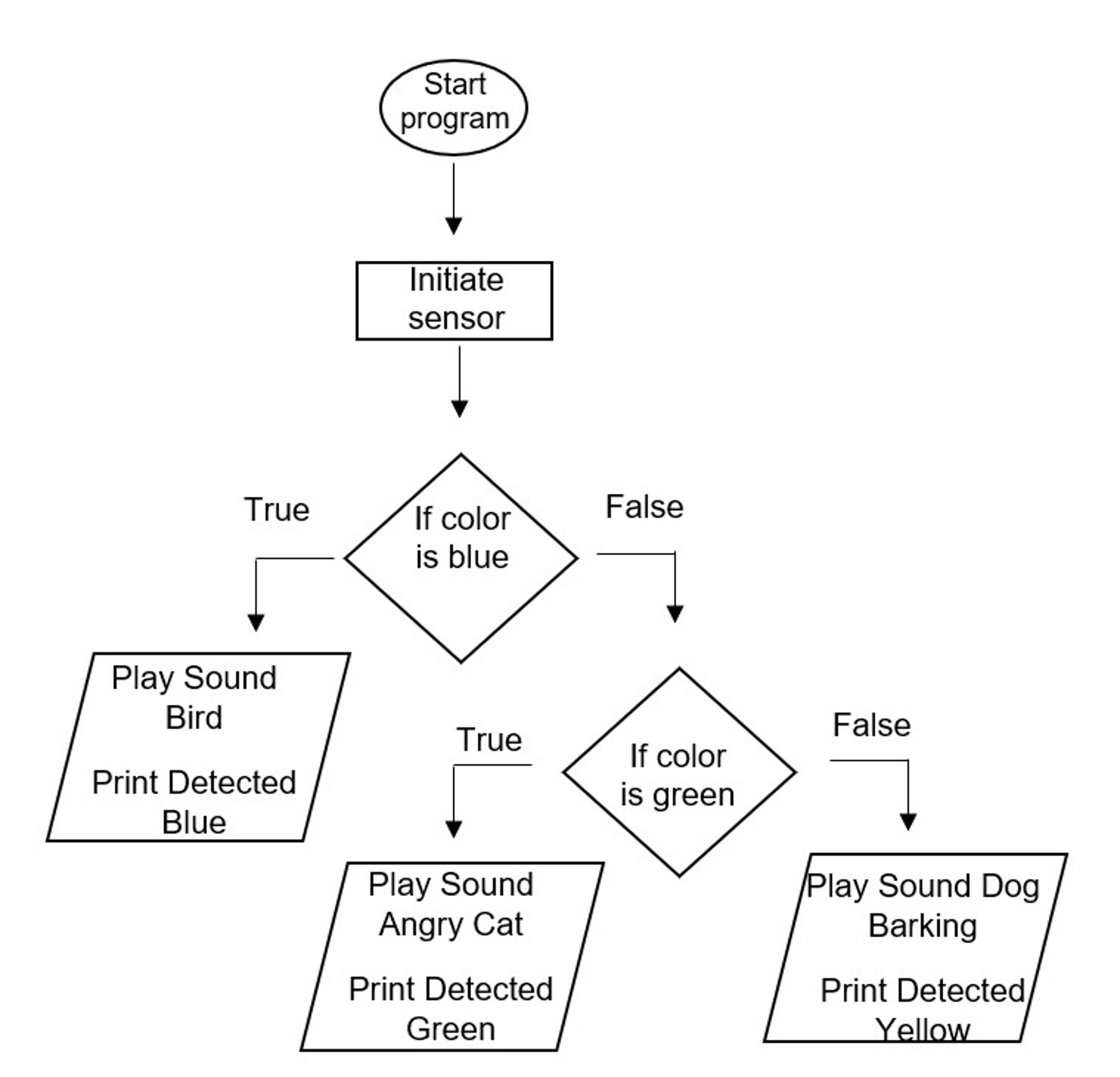
Ask students to modify their program to include an elif statement. Students should recognize that the elif should be between the if of the first condition and be followed by the else of the condition set by the elif.
Sample Program:
from hub import port
import runloop
import color_sensor
import color
from app import sound
async def main():
#if the color sensor senses blue, it will play bird sound
if color_sensor.color(port.A) == color.BLUE:
await sound.play('Bird')
print('Blue Detected')
#if the color sensor senses green, it will play cat angry sound
elif color_sensor.color(port.A) == color.GREEN:
await sound.play('Cat Angry')
print('Green Detected')
#if the color sensor does not sense blue, it will play dog bark 1 sound
else:
await sound.play('Dog Bark 1')
print('What happened?')
runloop.run(main())
Allow students time to explore the program and change sounds. Ask students to share their programs.
Evaluate
(Group Exercise, 5 minutes)
Teacher Observation:
Discuss the program with students.
Ask students questions like:
• How did the if/elif/else program work?
• What happened when you added a loop to your conditional statement?
• Why would you want to include if/else and elif statements in programs?
Self-Assessment:
Have students answer the following in their journals:
• What did you learn today about using conditional statements and loops together?
• What characteristics of a good teammate did I display today?
• Ask students to rate themselves on a scale of 1-3, on their time management today.
• Ask students to rate themselves on a scale of 1-3, on their materials (parts) management today.
Teacher Support
Students will:
• Program the color sensor using conditional code.
• Create a game.
• SPIKE Prime sets ready for student use
• Devices with the SPIKE App installed
• Student journals
CSTA
2-CS-02 Design projects that combine hardware and software components to collect and exchange data.
2-AP-10 Use flowcharts and/or pseudocode to address complex problems as algorithms
2-AP-13 Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
2-AP-16 Incorporate existing code, media, and libraries into original programs, and give attribution.
2-AP-17 Systematically test and refine programs using a range of test cases.
2-AP-19 Document programs in order to make them easier to follow, test, and debug.