Bike Riding for Data
Investigate using mathematical functions in a program
Questions to investigate
• How can we use mathematical functions in our program?
Prepare
• Ensure SPIKE Prime hubs are charged, especially if connecting through Bluetooth.
Engage
(Group Discussion, 5 minutes)
Engage students in a conversation about riding a bicycle as a mode of transportation.
Ask students to specifically talk about riding a bicycle to get from place to place, making sure to talk about the pros and cons of bicycle transportation.
Discuss places you might ride a bike, especially places that might make more sense to ride a bike than walk or take another type of transportation. Prompt students with examples if needed.
Explore
(Small Groups, 20 minutes)
Students will investigate riding a bicycle as a mode of transportation.
Direct students to the BUILD section in the SPIKE App. Here students can access the building instructions for the Smart Bike model. Ask students to build the model. The building instructions are also available at https://education.lego.com/en-us/support/spike-prime/building-instructions.
Direct students to open a new project in the Python programming canvas. Ask students to erase any code that is already in the programming area. Students should connect their hub.
Prompt students to think about how they program their Smart Bike to move. Students should write a program that allows the bike to move forward at a constant speed.
Sample Program:
from hub import port
import runloop
import motor_pair
async def main():
#pair motors
motor_pair.pair(motor_pair.PAIR_1, port.C, port.E)
#move 720 degrees, steering straight, at a velocity of 250
await motor_pair.move_for_degrees(motor_pair.PAIR_1, 720, 0, velocity=250)
runloop.run(main())
Moving with Math
Once students have their bike moving, challenge them to add to their program to change the velocity of their bike to move faster and slower. However, they should not just add new movements to the program. Instead, students should use variables and math functions to change the velocity.
Introduce the following math functions that can be used. These should all be common and familiar to students. Remind students that the order of operations will be followed in complex lines of math code.
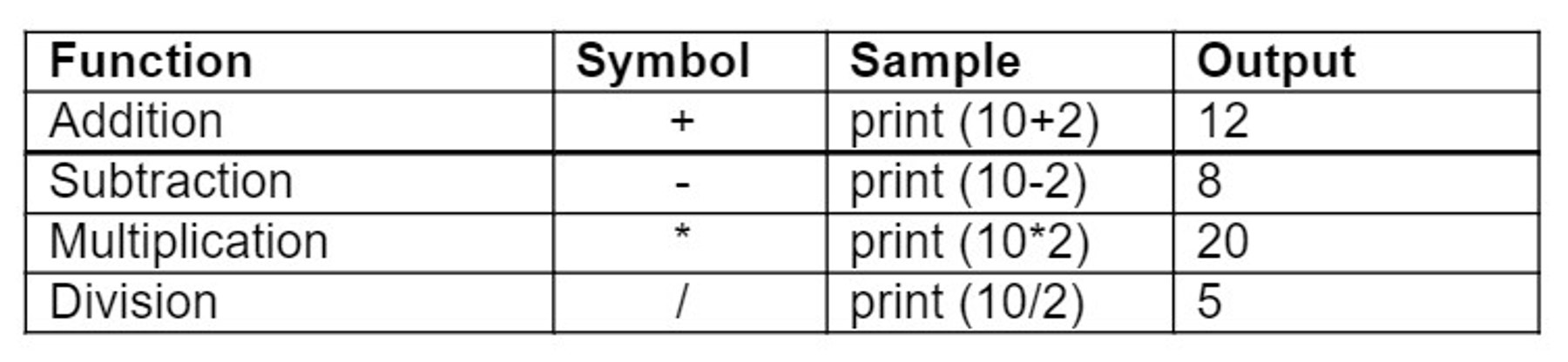
Using these math functions, students should be able to create a program that will increase and decrease the velocity of the bike.
Sample Program:
from hub import port, light_matrix, button
import runloop
import motor_pair
async def ride(bike_velocity):
#defines the ride function to write the velocity on the hub and move the bike farward
await light_matrix.write(str(bike_velocity))
await motor_pair.move_for_degrees(motor_pair.PAIR_1, 720, 0, velocity=bike_velocity)
async def main():
bike_velocity = 250
#pair motors
motor_pair.pair(motor_pair.PAIR_1, port.C, port.E)
while True:
#if the left button is pressed the velocity is reduced by 50
if button.pressed(button.LEFT):
bike_velocity -= 50
await ride(bike_velocity)
await runloop.sleep_ms(2000)
#if the right button is pressed the velocity is increaded by 50
if button.pressed(button.RIGHT):
bike_velocity += 50
await ride(bike_velocity)
await runloop.sleep_ms(2000)
runloop.run(main())
Allow students time to explore the program and try different values to add and subtract. Note that in line 15 you find this code: await light_matrix.write(str(bike_velocity))
The str before the bike_velocity tells the program to cast the integer to a string to allow it to be written on the hub. Casting is a way to convert data from one type to another in a particular situation. In this program, it doesn’t permanently change the data type, it converts it just to write on the hub. The variable is still an integer.
Explain
(Whole Group, 5 minutes)
Discuss with students how the program worked.
Ask students questions like:
• How can we use math functions in our program?
• How did using the math functions change the way your program worked?
• What is the benefit of controlling the speed with a variable?
The function ride was created to allow the same code to be run in two different places in the program. Creating functions helps to keep the program length at a minimum and it also allows us to make our program more efficient.
Elaborate
(Small Groups, 10 minutes)
Allow students to continue modifying their program to try new math functions based on what was shared from other students.
Challenge students to try adding new functions to their program to see how it changes the movements. Ask students to share their programs.
Sample program:
from hub import port, light_matrix, button
import runloop
import motor_pair
async def ride(bike_velocity):
#defines the ride function to write the velocity on the hub and move the bike farward
await light_matrix.write(str(bike_velocity))
await motor_pair.move_for_degrees(motor_pair.PAIR_1, 720, 0, velocity=bike_velocity)
async def main():
bike_velocity = 250
#pair motors
motor_pair.pair(motor_pair.PAIR_1, port.C, port.E)
while True:
#if the left button is pressed the velocity is reduced by 50
if button.pressed(button.LEFT):
bike_velocity /= 2
await ride(int(bike_velocity))
await runloop.sleep_ms(2000)
#if the right button is pressed the velocity is increaded by 50
if button.pressed(button.RIGHT):
bike_velocity *= 2
await ride(bike_velocity)
await runloop.sleep_ms(2000)
runloop.run(main())
Allow time for students to explore the program and use different math functions. Note: When a number is divided it automatically gets converted to a float, a decimal number. The velocity parameter must be an integer. This requires the casting of an integer for the velocity parameter.
Evaluate
(Group Exercise, 5 minutes)
Teacher Observation:
Discuss the program with students.
Ask students questions like:
• What happened when we added mathematical functions to our program?
• What did you learn about programming mathematical functions?
• What issue was discovered when division was used?
• Why was a motor pair used when there is only one motor moving the bicycle?
• What iterations can be made to our program?
Self-Assessment:
Have students answer the following in their journals:
• What did you learn today about using math functions in your program?
• What characteristics of a good teammate did I display today?
• Ask students to rate themselves on a scale of 1-3, on their time management today.
• Ask students to rate themselves on a scale of 1-3, on their materials (parts) management today.
Teacher Support
Students will:
• Program a bike model to move forward at a constant speed
• Create a program increase and decrease the speed of the bike model using math functions
• SPIKE Prime sets ready for student use
• Devices with the SPIKE App installed
• Student journals
CSTA
2-CS-02 Design projects that combine hardware and software components to collect and exchange data.
2-AP-10 Use flowcharts and/or pseudocode to address complex problems as algorithms
2-AP-13 Decompose problems and subproblems into parts to facilitate the design, implementation, and review of programs.
2-AP-16 Incorporate existing code, media, and libraries into original programs, and give attribution.
2-AP-17 Systematically test and refine programs using a range of test cases.
2-AP-19 Document programs in order to make them easier to follow, test, and debug.